Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial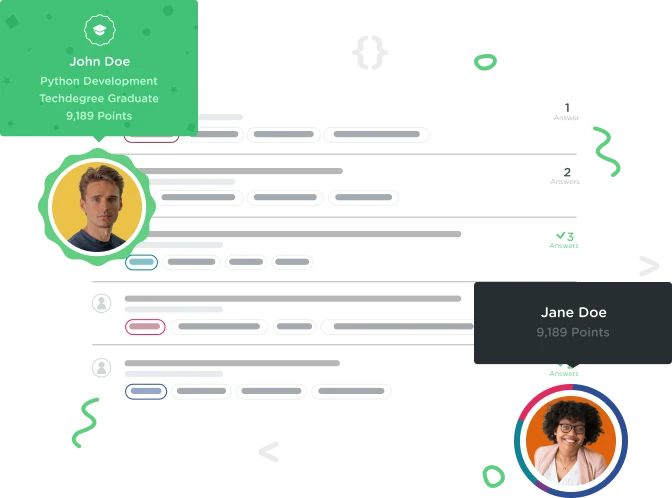
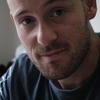
Gareth Partridge
13,421 PointsCurrently, the event listener applies a yellow background color to the section element and its child elements when click
Currently, the event listener applies a yellow background color to the section element and its child elements when clicked. Add a condition that changes the background of the <input> elements only.
I honestly have no idea on this one, any suggestions ?
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => {
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>JavaScript is an exciting language that you can use to power web servers, create desktop programs, and even control robots. But JavaScript got its start in the browser way back in 1995.</p>
<hr>
<p>Things to Learn</p>
<ul>
<li>Item One: <input type="text"></li>
<li>Item Two: <input type="text"></li>
<li>Item Three: <input type="text"></li>
<li>Item Four: <input type="text"></li>
</ul>
<button>Save</button>
</section>
<script src="app.js"></script>
</body>
</html>
6 Answers
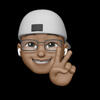
Allan Collado
10,248 Pointslet section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => {
if(e.target.tagName === 'INPUT'){
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
}
});

Alan Lane
9,156 PointsWOW! I got lucky, this is the solution that I finally got to work:
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => { if(e.target.tagName == "INPUT") { e.target.style.backgroundColor = 'rgb(255, 255, 0)'; } });

Flora Yin
6,792 PointsCould someone please explain why "INPUT" is needed here instead of "input"?
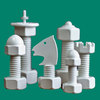
Steven Parker
231,268 PointsAs I told "anywayiam", you should always start a fresh new question instead of posting one as an "answer".
But quoting from the MDN page on tagName:
For DOM trees which represent HTML documents, the returned tag name is always in the canonical upper-case form. For example,
tagName
called on a<div>
element returns"DIV"
.
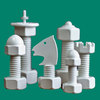
Steven Parker
231,268 PointsYou'll need to enclose the color assignment in a condtional ("if") statement. The "if" should check the tagName
attribute of the clicked item (e.target
) to see if it is an "INPUT".

anywayiam
3,970 PointsI have the same problem. It doesn't work.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section id='section'>
<h1>Making a Webpage Interactive</h1>
<p>JavaScript is an exciting language that you can use to power web servers, create desktop programs, and even control robots. But JavaScript got its start in the browser way back in 1995.</p>
<hr>
<p>Things to Learn</p>
<ul>
<li>Item One: <input type="text"></li>
<li>Item Two: <input type="text"></li>
<li>Item Three: <input type="text"></li>
<li>Item Four: <input type="text"></li>
</ul>
<button>Save</button>
</section>
</body>
</html>
let section = document.getElementById('section');
section.addEventListener('click', (event) => {
if(event.target.tagName == 'INPUT') {
event.target.style.backgroundColor = 'rgb(255, 255, 0)';
}
});
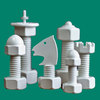
Steven Parker
231,268 PointsIn future, always start a fresh new question instead of posting one as an "answer".
But for this challenge you only need to add a conditional to the event handler. You should not make any changes to the other code including the HTML portion.

Sean Gibson
38,363 PointsI was running around in circles for a while on this one and found that my mistake was typing "tagname" instead of "tagName". Just in case this may help someone
Safae Merigh
9,827 PointsSafae Merigh
9,827 PointsWhy do we need "===" instead of just "=="?
Max van den Berge
6,506 PointsMax van den Berge
6,506 Pointsthis worked, i was struggling to :)