Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial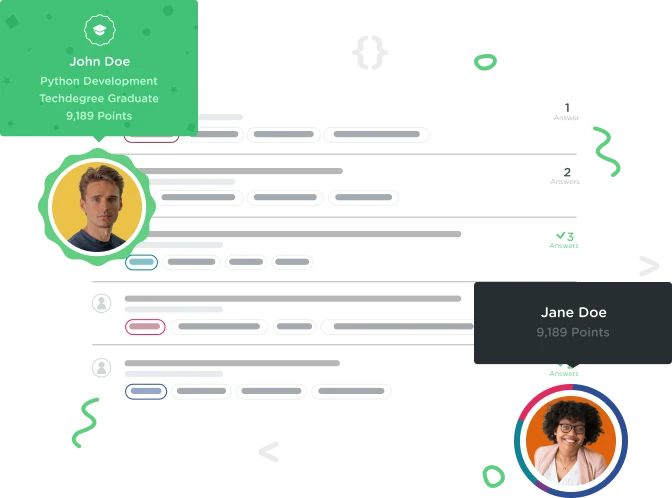
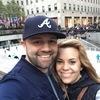
Janson Roberts
5,071 PointsCustom Initializers RGB
I'm told the following instructions:
In the editor, I've declared a struct named RGBColor that models a color object in the RGB space.
Your task is to write a custom initializer method for the object. Using the initializer, assign values to the first four properties. Using the values assigned to those properties, create a value for the description property that is a string representation of the color object.
For example, given the values 86.0 for red, 191.0 for green, 131.0 for blue, and 1.0 for alpha, each of the stored properties should hold these values and the description property should look like this:
"red: 86.0, green: 191.0, blue: 131.0, alpha, 1.0"
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red:Double, green: Double, blue: Double, alpha: Double){
self.red = 86.0
self.green = 191.0
self.blue = 131.0
self.alpha = 1.0
self.description = "red: \(self.red), green: \(self.green), blue: \(self.blue), alpha: \(self.alpha)"
}
}
I think I pretty much have it correct, but I keep getting errors! Either it tells me not to use the memberwise default, or it tells me to make sure the string I'm assigning the description property matches the example. Is there something clear that I'm missing here???
3 Answers
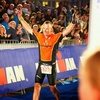
Steve Hunter
57,712 PointsHi Janson,
The init
method receives the values for the first four stored properties. Don't then hard-code them inside init
. You want to say self.red = red
etc.
You can then use the passed-in values inside the string, rather than accessing the self
ones. But give both a try.
If that still doesn't fix it, copy a link to the challenge in here and I'll go through it properly.
Steve.
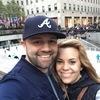
Janson Roberts
5,071 PointsThanks, Steve.
Right now, the code challenge is being unresponsive, so I'll take your word for it that this is correct. Perhaps I'll test it on the desktop later (currently using the app).
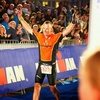
Steve Hunter
57,712 PointsLet me know how you get on.
Did you understand the point about the challenge not requiring values to be entered?
Steve.
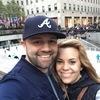
Janson Roberts
5,071 PointsHey Steve,
I finally was able to login to the desktop version here in Safari and test out the code. Worked like a charm. =)
It makes sense little by little each day. I understand now (I think) that the struct RGBColor has stored properties that were pre-listed in the editor by the instructor. After that, the instructions state that I have to create a custom initializer method and pass in the parameters for RGB (they're all of type Double). After that, I have to list the stored properties before an instance of the custom init method can be called. This means that for each variable, I have to assign a stored property, and since we're calling from outside the init method, I have to use the dot syntax. After I'm finished listing the values for the stored properties for the init method, I need to also do the same for the description property (represented in a String, because it's of type String). Finally (this step isn't required), I could call an instance of the method outside the scope of the struct that will actually be where I pass in the values for the RGB Doubles. (86.0 and so on...) Am I correct?
Thanks so much for explaining!
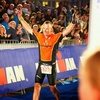
Steve Hunter
57,712 PointsHi Janson,
Yes, you pretty much have that down.
A struct contains stored properties. The init
method is called when an instance of the struct is required by the user/coder. The init
method must assign a value to all stored properties. Some of these values can be received by the init
method; in this case the red
, green
, blue
and alpha
values are received by init
, i.e. they are passed in by the user. The description
property is also assigned by the init
method but its value is not passed into the method.
The assignment inside the init
method (also known as a constructor in most other languages because that's what it does!) uses dot notation and the concept of self
. This is used to distinguish between the stored property, such as red
, and the passed-in value, also called red
. In Xcode, you can probably get away without using self
as long as you click on the correct version of red
but that's poor form and makes for unclear code - Java uses a similar way of distinguishing between these two variables but uses the keyword this
instead of self
. Same difference.
And, yes, you would create your instance of an RGBColor
by creating a new variable/constant to store it, then passing in the four required parameters into the RGBColor()
call. You would then be able to access all the stored properties, again, using dot notation. Using my example above, myColor
, accessing those properties would look like:
let redValue = myColor.red // redValue will hold 123.4
let greenValue = myColor.green // greenValue holds 12.34
let colorString = myColor.description // you get the idea! :-)
I hope that helps.
Steve.
Janson Roberts
5,071 PointsJanson Roberts
5,071 PointsHere's what I changed. I'm still not sure how I'm supposed to get back the double values if there's nowhere to input them???
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi there,
You're correct with that code - it should pass the challenge.
The
struct
is used by someone wanting to create their own specific RGB color. They would call thestruct
and pass in their chosen values to create a color. Theinit
method is called when astruct
is created so, on creation, the values are required. The values given in the question are just example ones - they aren't needing to be input anywhere.A new color can be created with:
But the challenge doesn't need a color to be created - it just wants the
struct
completing.Steve.