Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial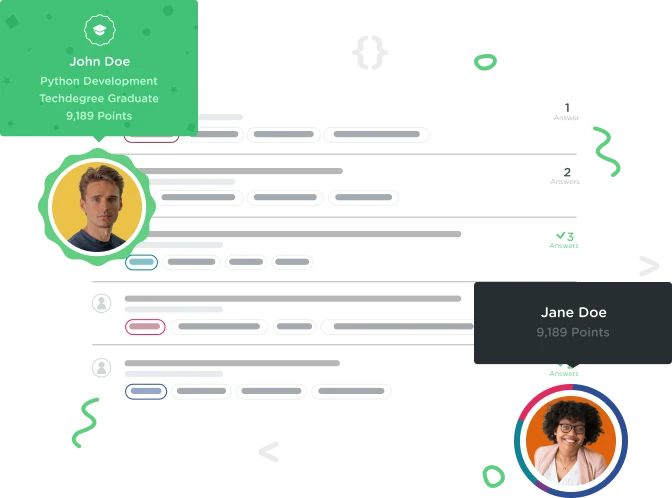
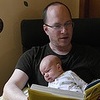
John Coffin
10,359 PointsCVM and other Practices
One of the reasons I started watching the videos here at Treehouse was because of a YouTube video of Ben Jakuben giving a brief summary of control-view-model. I thought that any place that teaches this (even as a concept worth thinking about) must be a class joint.
Jumping ahead to today, I am working on code for the forum contest. A work-around has led to code that is ... well ... awkward. I've listed some pseudo-code below that demonstrates my question in as simple a manner as possible. I would love to hear peoples opinions: With CVM, should the control get the information from the model and then the control updates the view (what I understood)? Or is it normal for the model update the view.
Cheers,
--- code below ---
I have a class clock (i.e. the model):
public class clock() {
private View mView;
private String mTime;
clock(View view) {
mView = view;
mTime = "12:00"
}
void updateDisplay() {
mView.setText(mTime);
}
}
The main activity (i.e. the control) initiates an update by calling mClock.updateDisplay():
public class MainActivity extends Activity {
private Clock mClock;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
View view = findViewById(R.id.view);
mClock = new Clock(view);
}
@Override
protected void onResume() {
super.onResume();
mClock.updateDisplay();
}
}
What I would prefer is if the clock (i.e. the model) did not own a copy of the view and did not update the view:
public class clock() {
private String mTime;
clock() {
mTime = "12:00"
}
String toString() {
return mTime;
}
}
This way, the main activity (the control) retrieves information from the clock (i.e. the model) and then it updates the view:
public class MainActivity extends Activity {
private View mView;
private Clock mClock;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mView = findViewById(R.id.view);
mClock = new Clock();
}
@Override
protected void onResume() {
super.onResume();
String time = mClock.toString();
mView.setText(time);
}
}
Make sense?
1 Answer

Ben Jakuben
Treehouse TeacherSorry for the delay - I'm just getting to posts I missed. What you have proposed makes sense from my perspective. Your Clock (model) object is responsible for holding the time. Your controller (MainActivity) asks it for the current time and then updates the View. Is that how you ended up implementing it?
The way I usually think about MVC when designing is to think about replacing the components down the road. In this proposal, you can easily replace any of the components with little or no updates to the others. You could potentially swap in a totally different Clock model and not have to update your View or Controller.
John Coffin
10,359 PointsJohn Coffin
10,359 PointsAny way we can move this from "General Discussion" to "Android ?"
PS Done.