Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial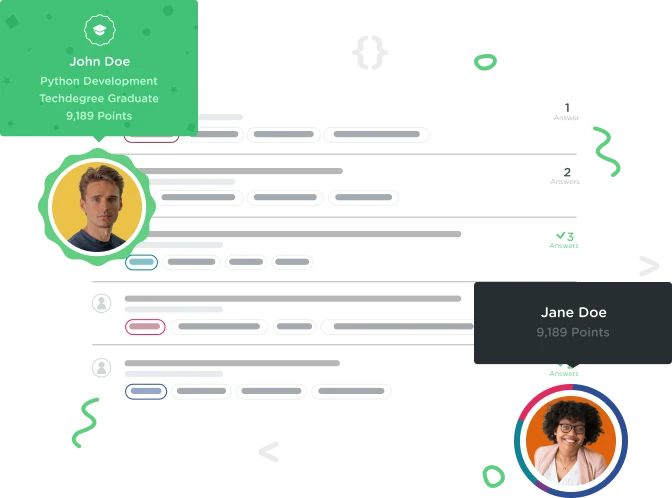

troy beckett
12,035 Pointscycle through images on gallery
Please take a look at my codepen:
http://codepen.io/tbeckett24/pen/EjxRdW
How would I go about making the large images cycle through in the same order they are in html when I click the right and left arrows.
So basically when I click the right arrow I want to go the next image in the html, when I click the left arrow its goes to the prev image in the html.
Any advice is appreciated however small. Im thinking It's going to involve an array and the each() function. But because my jquery skills are limited I find it hard to start off
5 Answers

Mark Buckingham
5,574 PointsHi Troy,
thanks for posting this I found it a good exercise for myself to solve this problem as I am learning these web technologies also.
Here is my solution, hope it helps:
HTML Changes - I added the image and paragraph elements to the HTML file. They will always be used so no need to dynamically add them as you were.
<div class="large-image">
<div class="thumb-large">
<img src=""></img>
</div>
<div class="thumb-large-descrip">
<p></p>
</div>
</div>
CSS Changes - new selector added:
.thumb-large img {
width: 500px;
height: 400px;
}
Javascript - various changes to make the code more modular but as you said the main idea is to store the image data in an array of objects, this makes navigation and lookup a lot easier. In this case I loaded the array from the thumbnails in the HTML, taking this a step further the array could be loaded from a data store such as a database or file allowing the thumbnails to be also loaded from the array.
// var images = [
// {source:"http://s24.postimg.org/vb31y1l1h/dotted_pic.jpg", caption:"dotty dotyy"},
// {source:"http://s7.postimg.org/hjzet1w7v/purple_pic.jpg", caption:"purple purple"},
// {source:"http://s2.postimg.org/oiepouf9l/gold_pic.jpg", caption:"gold gold"},
// {source:"http://s17.postimg.org/3q6eww7tb/splashy_pic.jpg", caption:"splashy"}
// ];
var images = [];
var imageIndex = 0;
var $mainImage = $(".thumb-large img");
var $mainCaption = $(".thumb-large-descrip p");
var $thumbnails = $(".thumbnails img");
function showImage(index) {
$mainImage.attr({src: images[index].source});
$mainCaption.text(images[index].caption);
}
function findImageIndex(imageSource) {
for(var i = 0; i < images.length; i += 1) {
if (images[i].source === imageSource) {
return i;
}
}
return -1;
}
// Load array with image data from html.
var imageCount = $thumbnails.length;
for(var i = 0; i < imageCount; i += 1) {
images.push({
source: $thumbnails[i].src,
caption: $thumbnails[i].alt
});
}
// Show initial image.
showImage(imageIndex);
// Handle click on thumbnail image.
$(".thumb-image").click(function() {
imageIndex = findImageIndex($(this).attr("src"));
showImage(imageIndex);
});
// Handle left arrow click.
$(".left-arrow").click(function() {
if (imageIndex === 0) {
imageIndex = images.length -1;
}
else {
imageIndex -= 1;
}
showImage(imageIndex);
});
// Handle right arrow click.
$(".right-arrow").click(function() {
if (imageIndex === images.length -1) {
imageIndex = 0;
}
else {
imageIndex += 1;
}
showImage(imageIndex);
});
thanks, Mark

troy beckett
12,035 PointsThanks alot for helping me out, I really really appreciate what you shown me there I going to go away and learn if I have some trouble with it I'll let you know. Because obviously i more worried about understanding it fully

troy beckett
12,035 PointsCould you explain what the return -1 does in the function findimageindex()?

Mark Buckingham
5,574 PointsI included as a return result in cases where no match is found, it is not needed in this example and is a coding habit I have from developing in c#. It can be removed altogether as JavaScript returns undefined when the code returns nothing.
Mark.

troy beckett
12,035 PointsOk, thanks.