Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial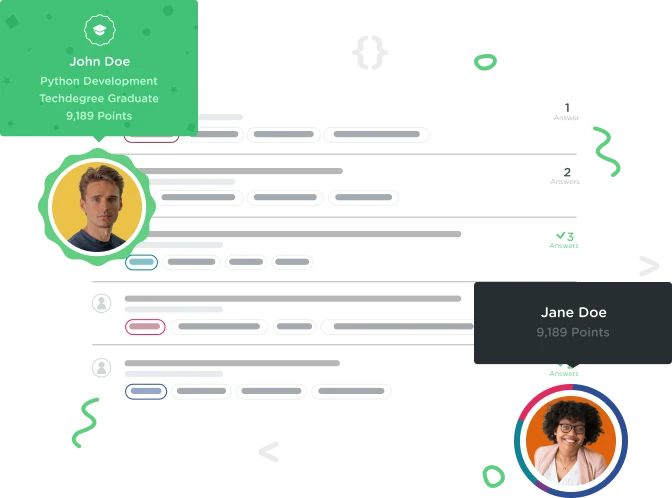

Herman Vicens
12,540 PointsData mismatch problems
I've been trying to fix this problem in many ways unsuccessfully. Please give me an idea how to fix it.
thanks,
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int count = 0;
double acum = 0.0;
foreach ( int x in frogs )
{
acum = acum + Convert.ToDouble(frogs[count].tongueLength);
count +=1;
}
return acum / count;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
2 Answers
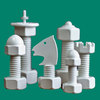
Steven Parker
231,275 PointsIt looks like you have the right idea, but a few issues:
- if you iterate through frogs each item will be a Frog, not an int
- you won't need to index into frogs since you will have an individual Frog item in the loop
- you don't need to convert the lengths into doubles to add them to the accumulator
- the property name is "TongueLength" (with a capital "T")
UPDATE: This type of loop ("foreach") does not give you an index like a "for" loop would. Instead, it gives you one of the items from the collection. So instead of "int x in frogs
" you might write "var frog in frogs
". Then instead of "frogs[x].TongueLength
" you might have "frog.TongueLength
"

Herman Vicens
12,540 PointsThanks Steve. It seems that I am almost done but I ran out of ideas with getting to the TongueLength property. I am getting a CS0020 error and I tried everything that my limited C# knowledge permits. Can you be more specific with the Frog and frogs in the loop? In the for loop excersise, the frogs[x].TongueLength worked. Not working in the foreach loop and don't know why?
Thanks,
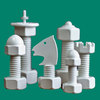
Steven Parker
231,275 PointsYou could re-write the challenge using a "for" loop, but I assumed you wanted to use "foreach" instead. It's a good choice, and I added an update to my answer above.
If you're still having trouble, show the code that you have at this point and I'll take another look.