Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial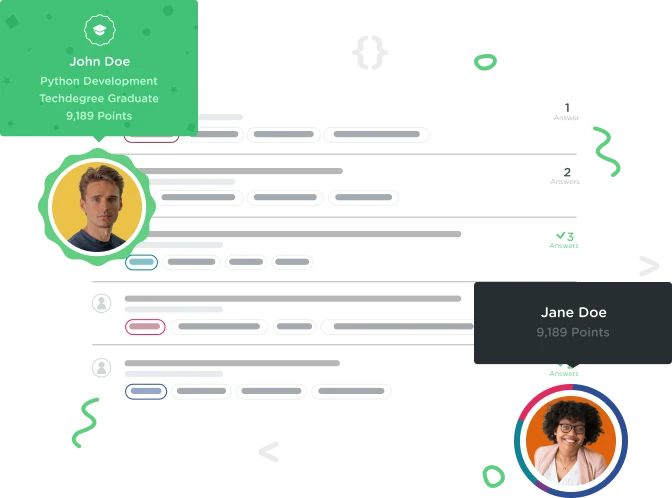

Aftar Khan
129 PointsData Structures and Algorithms?
What is Data Structures and Algorithms. Are they hard to learn I heard that to get a job as a programmer your data structure must be strong. What that actually mean. I am learning JavaScript and i don't find any course on them.
2 Answers

Alexander La Bianca
15,959 PointsAn Array is fundamental data structure. A Hash Table is another. Now you can search,sort, copy etc values of those data structures by applying search or sort algorithms on them. The performance or complexity of these algorithms can be measured using Big O notation.
Since you are learning javascript here is an example of searching an an array without any built in functions. This algorithm is Big O(n) where n is the size of the array. The performance is linear to the size of the array
function find5(array) {
for(var i = 0; i<array.length;i++) {
if(array[i] === 5) {
return true;
}
}
return false;
}
let arr = [1,2,3,4,5,6,7,8,9,10];
find5(arr) //Big O(n)
If the array has 1000 or 10000 items in it. The performance of this algorithm directly depends on the size of the array. Which is why Big O is O(n).
Another example is Big O(1) which means the performance is constant. Regardless of the size of the input.
function getFirstItem(array) {
return array[0];
}
let arr = [1,2,3,4,5,6,7,8,9,10];
getFirstItem(arr); //Big O(1)
The peformance is constant. Regardless if the array contained 1000 or 100 000 000 items.
This all ties back to Data Strucutures (the array in this example) and algorithms (the mechanism that is applied on the data structure).

Aftar Khan
129 PointsThanks sir, I got some help then it means that data structure and algorithms are organizing data and performing actions to them. Also mean that its all about logics am i right? And also if so then data structure and algorithms are same in all languages right? Please answers these questions sir.

Alexander La Bianca
15,959 Pointsyou are correct