Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial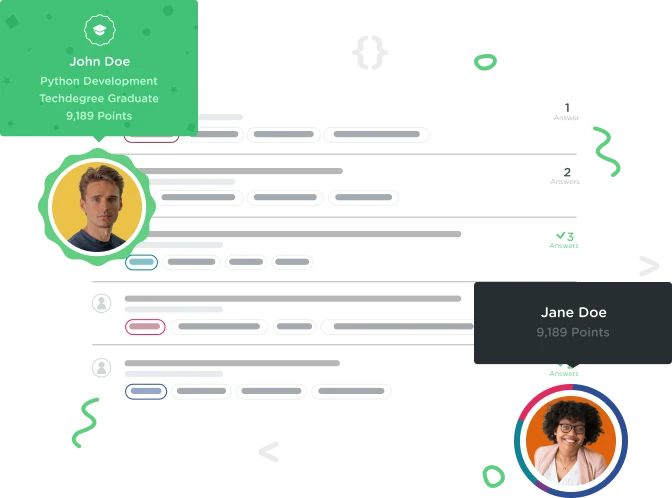
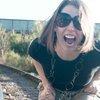
Beth Singley
3,491 Pointsdatabase rows not updating
Hi, I have the lesson copied out in sublime text and am able to create tables and rows using this code. However, when I change the dictionary point values, save, and rerun the file through the terminal, the database is not updating with the new points values.
Interestingly...when I go into mysqlworkbench and drop the table, I can get the python to create a new table with the right users and vales. Obviously there's something wrong in the except block. Help?
Here's my code.
from peewee import *
from playhouse.db_url import connect
#db = MySQLDatabase('students.db')
db = connect('mysql://root:Purple12!@localhost:3306/python_work')
class Student (Model):
username = CharField(max_length=255, unique=True)
points = IntegerField(default=0)
class Meta:
database = db
students = [
{'username': 'kennethlove',
'points': 888},
{'username': 'oscargrouch',
'points': 8000},
{'username': 'jokenston2',
'points': 7346},
{'username': 'twoheadedcow',
'points': 1983},
{'username': 'giantman',
'points': 250}
]
def add_students():
try:
for student in students:
Student.create(username=student['username'],
points=student['points'])
except IntegrityError:
student_record = Student.get(username=student['username'])
student_record.points = student['points']
student_record.save()
def top_student():
student = Student.select().order_by(Student.points.desc()).get()
return student.username
if __name__ == '__main__':
db.connect()
db.create_tables([Student], safe = True)
add_students()
print("Our top student right now is: {}." .format(top_student()))
1 Answer
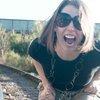
Beth Singley
3,491 PointsI found it!!
The progression through the dictionary was inside the try block. Updated (working!) code is as follows:
def add_students():
for student in students:
try:
Student.create(username=student['username'],
points=student['points'])
except IntegrityError:
student_record = Student.get(username=student['username'])
student_record.points = student['points']
student_record.save()