Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial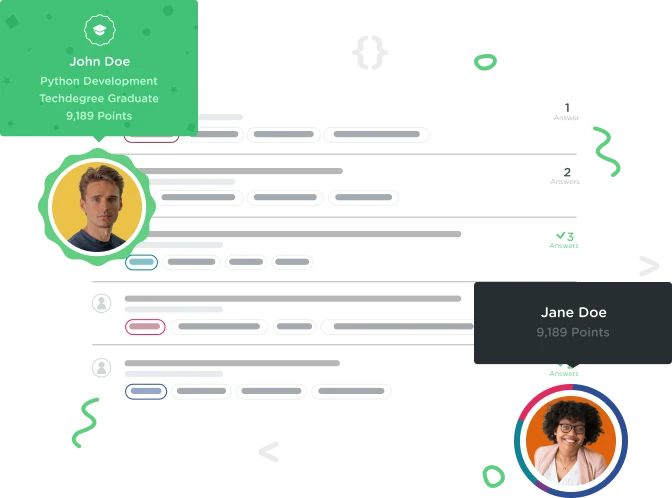

Björn Norén
9,569 Pointsdatareceived event will not fire, help?
Hello there, I'm practising working with a Arduino through bluetooth there I get a connection but my program seems to not receive any data. I have followed the instructions from Microsoft page: https://msdn.microsoft.com/fi-fi/library/system.io.ports.serialport.datareceived(v=vs.110).aspx?cs-save-lang=1&cs-lang=csharp#code-snippet-1 but the function "DataReceivedHandler" is not firing since it won't write out the text: "Data Received". This has been a unsolving problem some days so any help is very much appreciated!
Best regards, Björn
using System;
using System.Collections.Generic;
using System.IO.Ports;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace PortDataReceived
{
class PortDataReceived
{
public static void Main(string[] args)
{
SerialPort mySerialPort = new SerialPort("COM5");
mySerialPort.BaudRate = 9600;
mySerialPort.Parity = Parity.None;
mySerialPort.StopBits = StopBits.One;
mySerialPort.DataBits = 8;
mySerialPort.Handshake = Handshake.None;
mySerialPort.RtsEnable = true;
mySerialPort.Open();
mySerialPort.DataReceived += new SerialDataReceivedEventHandler(DataReceivedHandler);
Console.WriteLine("Press any key to continue...");
Console.WriteLine();
Console.ReadKey();
mySerialPort.Close();
}
private static void DataReceivedHandler(
object sender,
SerialDataReceivedEventArgs e)
{
SerialPort sp = (SerialPort)sender;
string indata = sp.ReadExisting();
Console.WriteLine("Data Received:");
Console.Write(indata);
}
}
}
1 Answer
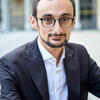
Christian ROLLET
11,252 PointsFrom the link you posted, we can see
Data events can be caused by any of the items in the SerialData enumeration. Because the operating system determines whether to raise this event or not, not all parity errors may be reported. The DataReceived event is also raised if an Eof character is received, regardless of the number of bytes in the internal input buffer and the value of the ReceivedBytesThreshold property.
So maybe you did not receive an Eof character. You should wait a little and check content of ReceivedBytesThreshold. If ReceivedBytesThreshold = 0, it means you do not receive anything. If not, it means operating system decides not to raise DataReceived (probably because there is no EoF character.
Something like
mySerialPort.DataReceived += new SerialDataReceivedEventHandler(DataReceivedHandler);
// Ugly way to pause the program
Console.WriteLine("Press any key to check ReceivedBytesThreshold...");
Console.ReadKey();
Console.WriteLine (mySerialPort.ReceivedBytesThreshold);
Console.WriteLine("Press any key to continue...");
Console.WriteLine();
Also you can try to handle ErrorReceived error.
Hope this help