Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial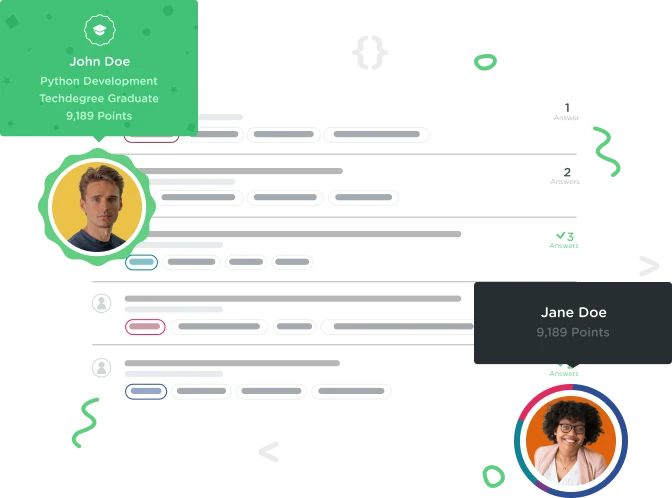
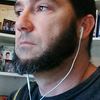
richard maxon
3,089 Pointsdate formatter: weather app
code in CurrentWeather.java
~snip~
public String getFormattedTime(){
SimpleDateFormat formatter = new SimpleDateFormat("h:mm a");
formatter.setTimeZone(TimeZone.getTimeZone(getTimeZone()));
Date dateTime = new Date(getTime() * 1000);
String timeString = formatter.format(dateTime);
return timeString;
}
~snip~
Date dateTime = new Date(getTime() * 1000); // errors getTime cant be applied to long.
Java compile provides error: CurrentWeather cannot be applied to given types; Date dateTime = new Date(getTime() * 1000); ^ required: long found: no arguments reason: actual and formal argument lists differ in length 1 error
10 Answers
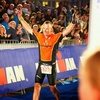
Steve Hunter
57,712 PointsSorry for the quick answer earlier - I was on my way out; back now!
Yes, your getter for temperature
isn't quite right.
That would look like:
public double getTemperature() {
return mTemperature;
}
You call the get methods when you want to, erm, get the property that they access; in this case, temperature
. This means you can nicely hide your instance variables by making them private
and the outside world can only get to them by using the getter or setter methods. That means you'll pass a parameter to a setter methods but not to a getter. Similarly, a setter method returns nothing, void
, but the getter returns the value from within the dark depths of the class.
Your next line of code is also a little out. You are trying to pass in the data from the JSON object. You need to call the setter method as you are setting the time inside the instance of CurrentWeather called currentWeather
.
You need to change that to:
currentWeather.setTime(currently.getLong("time"));
using setTime(long time)
. The JSON value for the key "time" is a long
so the getLong
method is used to get the number value corresponding to the time key.
I hope that makes sense!!
Steve.
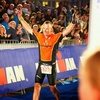
Steve Hunter
57,712 PointsHiya,
I've edited the code to make it look nicer.
Your getTime()
method is taking a parameter; it shouldn't as it just returns one (it is a getter method).
If you amend your getTime
method to be public long getTime()
that should do the trick!
Steve.
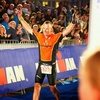
Steve Hunter
57,712 PointsHi Richard,
That all looks OK, oddly! Can you share your getTime()
method, please? Also, show us the line where you created the mTime
variable.
Thanks
Steve.
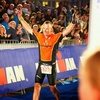
Steve Hunter
57,712 PointsYour getTime()
method should be pretty simple:
public long getTime(){
return mTime;
}
And mTime
should be created at the top of the class like:
private long mTime;
Are yours the same/similar?
Steve.
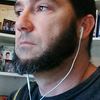
richard maxon
3,089 Pointshere they are. I thought looked correct.
public class CurrentWeather {
private String mIcon;
private long mTime;
private double mTemperature;
private double mHumidity;
private double mPrecipChance;
private String mSummary;
private String mTimeZone;
.
.
.
.
.
.
public long getTime(long time) { /// <-- this is taking a parameter which is why your code is expecting a 'long'
return mTime;
}
public void setTime(long time) {
mTime = time;
}
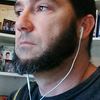
richard maxon
3,089 Pointspublic class CurrentWeather {
private String mIcon;
private long mTime;
private double mTemperature;
private double mHumidity;
private double mPrecipChance;
private String mSummary;
private String mTimeZone;
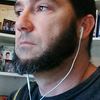
richard maxon
3,089 Pointsi dont' know why the code snipet wont paste correctly :( \
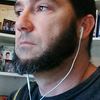
richard maxon
3,089 PointsSteve - that did the trick.
rich
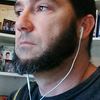
richard maxon
3,089 Pointssso is this incorrect?
public double getTemperature(double temperature) { <------since this is taking a parameter?
return mTemperature;
}
turns out "Long" is giving me another error.
currentWeather.getTime(currently.getLong("time")); <----give error "getTime in CurrentWeather can't be applied to 'long'.
If fixed the error above and cleaned the proejct.
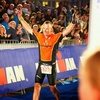
Steve Hunter
57,712 PointsYeah, that's also not quite right.
Getters just return a value, they don't take one. So, they have () at the end of their declaration.