Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial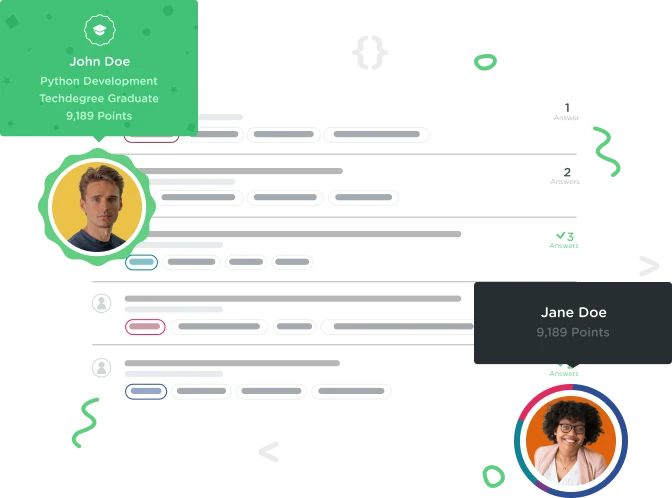
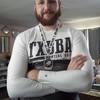
Zach Rebstock
4,632 PointsDate symbol cannot be found
import com.teamtreehouse;
import java.util.Date;
public class Treet {
private String mAuthor;
private String mDescription;
private Date mDate;
public Treet(String author, String descr, Date date) {
mAuthor = author;
mDescription = descr;
mDate = date;
}
public getAuthor() {
return mAuthor;
}
public getDescription() {
return mDescription;
}
public getDate() {
return mDate;
}
}
import com.teamtreehouse.Treet;
public class Example {
public static void main(String[] args) {
Treet treet = new Treet("Joe Smith", "This is a new post about stuff", new Date(1505140569));
System.out.printf("The tweet is: %s %n", treet);
}
}
symbol: class Treet
location: class Example
Example.java:8: error: cannot find symbol
Treet treet = new Treet("Joe Smith", "This is a new post about stuff", new Date(1505140569));
^
symbol: class Date
location: class Example
4 errors
2 Answers

pluboj
5,954 PointsHi Zach,
You may need to import Date to your Example class just like you did in the Treet class.
import java.util.Date;

Marcin Lubke
13,253 PointsI have the same problem.
I've already found out that I have to import java.util.Date also in my Example.java file, but I'm not sure why you have to do this.
I thought that it works like in some other programming languages (for example C++ if I remember correctly) - if I import Treet.java, where util.Date is already imported I shouldn't have to import it again.

Wajid Mohammed
5,698 PointsI had the same issue just as mentioned here by Marcin and agree with him that once imported in the Treet class why should I need to re-import again in the Example main class. I could only think of the reason following reason below.
Since the scope of the Date and its usage is inside the Treet Class whereas in the main Example we are passing a new Date object. This new Date object need to be created in Example even before the Treet Object constructor is ready to receive the Date object to initialise it. This means Example must import Date class to create the new Date object before passing it off to the Treet.
If someone else has a some other explanation then please add your comment.
Thank you :)