Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial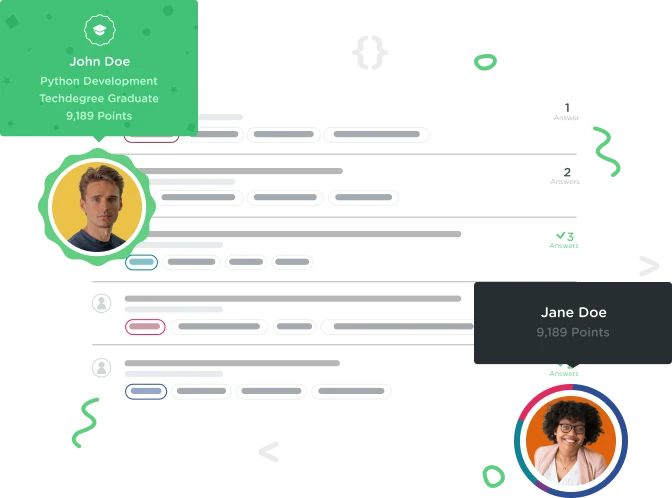
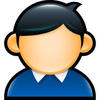
Daniel Hildreth
16,170 PointsDateTime C# Challenge 3
I need help figuring this out. When I preview my code I don't get any errors, but I check my work and it says "Did you forget to assign the TimeOfDay property?" What am I doing wrong here?
using System;
using System.IO;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
WeatherForecast weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
return weatherForecast;
DateTime timeOfDay;
if(DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
}
}
}
using System;
/* Sample CSV Data
weather_station_id,time_of_day,condition,temperature,precipitation_chance,precipitation_amount
HGKL8Q,06/11/2016 0:00,Rain,53,0.3,0.03
HGKL8Q,06/11/2016 6:00,Cloudy,56,0.08,0.01
HGKL8Q,06/11/2016 12:00,PartlyCloudy,70,0,0
HGKL8Q,06/11/2016 18:00,Sunny,76,0,0
HGKL8Q,06/11/2016 19:00,Clear,74,0,0
*/
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
public string WeatherStationId { get; set; }
public DateTime TimeOfDay { get; set; }
}
}
1 Answer

Chris Shaw
26,676 PointsHi Daniel,
Your code is almost correct, your return
statement is simply on the wrong line as it should be the last line in your function, see the below.
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
WeatherForecast weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
return weatherForecast;
}
}
}
Happy coding!
Philip Zayats
2,360 PointsPhilip Zayats
2,360 PointsWhat?
Program.cs(14,7): error CS0246: The type or namespace name
DateTime' could not be found. Are you missing
System' using directive?Program.cs(16,11): error CS0103: The name `DateTime' does not exist in the current context
Program.cs(18,37): error CS0841: A local variable `timeOfDay' cannot be used before it is declared