Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial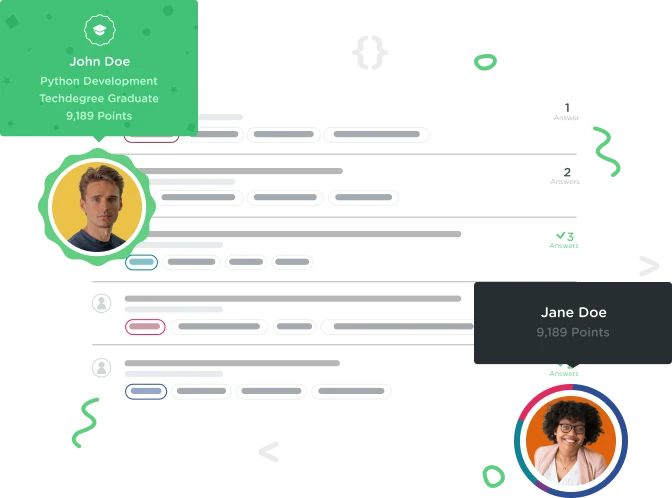

Izaak Prats
4,149 PointsDayAdapter Error "Attempt to invoke method '...' on a null object reference"
So tapping on 7-Day crashes my app and this is the only error being thrown, but I didn't have this problem before just now checking if tapping works. So, I tried commenting out the code for accessing the tapping feature and it's still acting up. It's saying "Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference" for the line in DayAdapter that read
holder = (ViewHolder) convertView.getTag();
I don't know why this would be an issue, so I tried renaming one of my TextView's in daily_list_item to conform with what I had in DayAdapter thinking that was the issue, but nothing changed. When I try placing a debugger in the file that's throwing the error, it's not stopping and continues to crash regardless. So, my question is does anyone have an idea of what could be going wrong? I will post the codes on request, but if you could just give me an idea of why the debugger isn't stopping appropriately I might be able to fix it myself.
Edit: Here is my code for DayAdapter
package com.example.jeffrey.stormy.adapters;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.example.jeffrey.stormy.R;
import com.example.jeffrey.stormy.weather.Day;
public class DayAdapter extends BaseAdapter {
private Context mContext;
private Day[] mDays;
public DayAdapter(Context context, Day[] days) {
mContext = context;
mDays = days;
}
@Override
public int getCount() {
return mDays.length;
}
@Override
public Object getItem(int position) {
return mDays[position];
}
@Override
public long getItemId(int position) {
return 0; // not useful to us
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if(convertView == null) {
// brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
if (position == 0) {
holder.dayLabel.setText("Today");
} else {
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayLabel);
}
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
holder.dayLabel.setText(day.getDayOfTheWeek());
return convertView;
}
private static class ViewHolder {
ImageView iconImageView; // public by default
TextView temperatureLabel;
TextView dayLabel;
}
}
As for your questions about the debugging process, yeah, I'm making sure I click debugger, my breakpoints are working other places in the project, just not where I need them to. I went ahead to check to make sure my breakpoints were working and they are most certainly prior to the error that is thrown to the logcat. And I tried stepping over things line by line to see where the null object was, but I couldn't find anything, especially once it got to the inner workings of the Android library.
1 Answer
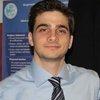
adamabdulghani
7,864 PointsHi Izaak,
Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference
Probably you aren't instantiating the TextView that you're trying to setText to, anyway it would be great if you can post the code here so that we could better help you. Concerning the debugger, are you making sure that you're pressing the Debug button (shift +F9) and not the Run button? also you might be adding the breakpoint in your code after the exception, so the app would crash before it reaches the breakpoint you added.
Izaak Prats
4,149 PointsIzaak Prats
4,149 PointsEdited to answer about the debugger and add my code for the DayAdapter.
adamabdulghani
7,864 Pointsadamabdulghani
7,864 PointsHello Izaak, it looks like you have to instantiate holder.dayLabel in the if(convertView == null) {} block.
Hope This helps and good luck ! :-)
Izaak Prats
4,149 PointsIzaak Prats
4,149 PointsThis wound up fixing what I was trying to do, I was neglecting to revert back some changes that had entailed, but after that, everything is running great! Now I can put this aside and move on to my next project (and probably course!)