Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial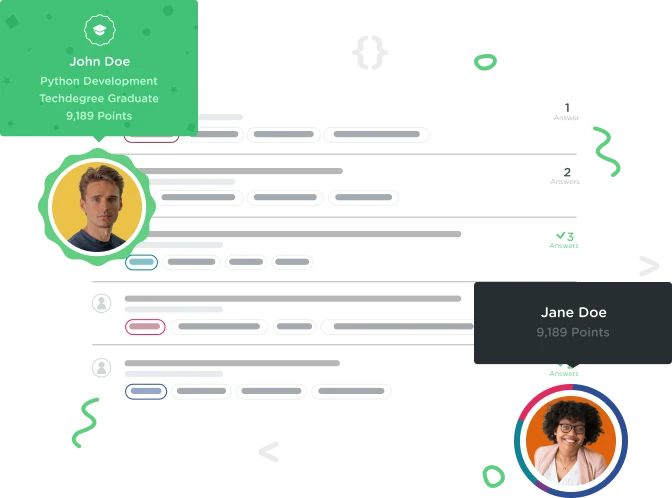

charlesdi
5,706 PointsdayAdapter not recognizing xml attributes.
while following the directions in the Android Lists and Adapters videos at the point where we create the dayAdapter.java file Android Studio starts to throw errors when I fill in the getView method.
In particular it does not seem to recognize
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
iconImageView, temperatureLabel, and dayLabel are not recognized and I can't seem to find the reason why.
On top of that, when I follow the directions Android Studio automatically creates another java file with the same code in it called "ViewHolder." Was something deprecated and I don't know about it?
Full code for dayAdapter.java file:
package com.treehouse.android.stormy.adapters;
import android.content.Context;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.treehouse.android.stormy.R;
import com.treehouse.android.stormy.weather.Day;
import org.w3c.dom.Text;
/**
* Created by charlesdirenzo on 8/1/17.
*/
//this will act as an adapter to pass information from the day object to the
public class dayAdapter extends BaseAdapter
{
private Context mContext;
private Day[] mDays;
public dayAdapter(Context context, Day[] days)
{
mContext = context;
mDays = days;
}
@Override
public int getCount()
{
return mDays.length;
}
@Override
public Object getItem(int position)
{
return mDays[position];
}
@Override
public long getItemId(int position)
{
return 0; //this will go unused. This can be used to tag items for easy reference.
}
@Override
public View getView(int position, View convertView, ViewGroup parent)
{
//viewHolder pattern will help us re-use resources and memory, power, and help the list be smoother.
//convert view is the view that we are reusing. if it is null it's new, and if not then it has data in it.
//layout inflater is an android object that takes xml layout and turns them into views in code that we can use.
RecyclerView.ViewHolder holder;
if (convertView == null)
{
//brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item,null);
holder = new RecyclerView.ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
convertView.setTag(holder);
}
else
{
holder = (RecyclerView.ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
holder.dayLabel.setText(day.getDayOfTheWeek);
}
return null;
}
private static class ViewHolder {
ImageView iconImageView;
TextView temperatureLabel;
TextView dayLabel;
}
}
2 Answers

Ben Jakuben
Treehouse TeacherI haven't seen that behavior yet myself, but I haven't created any new RecyclerViews in a while. That sounds like something changed with Android Studio. You should be able to delete the ViewHolder class that was generated and use your own, or set up the new one like we do in the video and use that.
Normally Java programs have a convention of having one class per file, so I can understand why the ViewHolder class got moved to its own file. I usually follow that convention, but in a few specific cases like this one I would put a small and directly related class in the same file as the other class where it is used.
Once you get your ViewHolder set up one way or another, all the error lines should appear as things will then be able to be resolved by the compiler.

Ben Jakuben
Treehouse TeacherThat often happens when there's an error somewhere in a resource file and the R class is unable to be generated. But there certainly are other reasons it could happen. It's hard to troubleshoot without a full view of your project. Can you either zip your code and email it to me at ben@teamtreehouse.com, or share it via a public repo on GitHub or elsewhere?
charlesdi
5,706 Pointscharlesdi
5,706 PointsI'm very close to making this work. Unfortunately, Android Studio says that it no longer recognizes 'layout' or 'id' in the findViewById statements. I've already done a clean and rebuild to no affect. Any idea what might be causing this? at first I thought it was faulty import statements.