Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial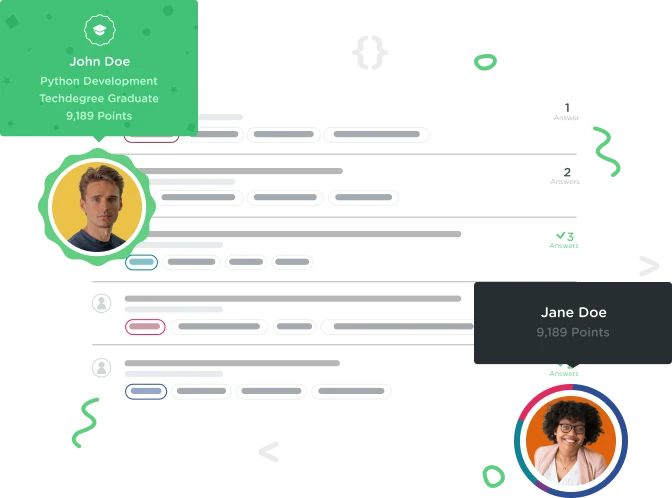
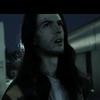
codeoverload
24,260 PointsDebugger: "Frame is not available"
When I set a break point in the getCurrentDetails method and I try to step out of it, the debugger says "Frame is not available" and stops/closes the app.
However, if I'm running the app normally, everything's fine.
MainAcitvity:
package com.codeoverload.stormy;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.widget.Toast;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "1fb52342ec84c2f2631b797ff48a6a03";
double latitude = 37.8267;
double longitude = -122.4233;
String forecastUrl = "https://api.darksky.net/forecast/" + apiKey +
"/" + latitude + "," + longitude;
Log.d(TAG, forecastUrl);
if (isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
e.printStackTrace();
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
} else {
alterUserAboutError();
}
}
catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
} else {
Toast.makeText(this, R.string.network_unavailable_message, Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main UI code is running!");
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON: " + timezone);
// BREAK POINT
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("temperature"));
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alterUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
It's not that big of a problem, but I would still like to know what's going on.
Thanks in advance!
2 Answers

jim vorous
225 Pointsthis answer is wrong it doesn't help at all

Gwinyai Nyatsoka
7,428 PointsWhen you get the message "frames not available" it means that no more frames are available for debugging. The frames are part of Android Studio's debugging which gives you access to the list of threads running in your application. It is the long list of processes you see in the debugging window. So what is happening is that Android Studio loses knowledge of the threads it had before you set the breakpoint when you are stepping out. This might be happening in your case because by default when you set a breakpoint, it stops execution of all threads. When you step out, the threads that follow rely on the threads before to work which is what closes the app. Since you are setting your breakpoint at getCurrentDetails, my best assumption is that it did not get the response from OKHttp in time since it is happening in a background thread. You can try two things to get it to work. First, try and right click on the breakpoint and change the breakpoint from pausing all threads to just that thread. This should allow OKHttp to get the response in time before being cut off. Or, shift your breakpoint further down and see if it works.