Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial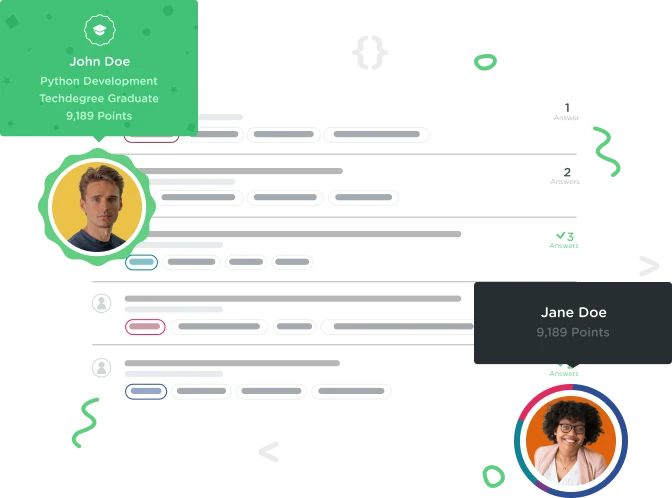

Timmie Nilsson
4,463 PointsDecipher or deserialize a String array.
Hi I am in the serializations Java Data project and in my workspace I only get back wierd coding when im reading them back from a file like this from consol:
Tweet: "Hej, har ni hört senaste nyheten?" by Timmie Nilsson on Fri Mar 23 12:03:19 UTC 2018
Tweet: "This is so fuuuun!" by journeytocode on Fri Mar 23 12:03:19 UTC 2018
[Lcom.dfsweb.Tweet;@2d98a335
[Lcom.dfsweb.Tweet;@2d98a335
As you can see it works fine when i make a for loop without saving them and reading them back from a file.
import com.dfsweb.Tweet;
import com.dfsweb.Tweets;
import java.util.Date;
import java.util.Arrays;
public class Example {
public static void main(String[] args) {
Tweet tweet = new Tweet(
"Timmie Nilsson",
"Hej, har ni hört senaste nyheten?",
new Date()
);
Tweet secoundTweet = new Tweet(
"journeytocode",
"This is so fuuuun!",
new Date()
);
Tweet[] tweetArr = {tweet, secoundTweet};
Arrays.sort(tweetArr);
for (Tweet exampleTweet : tweetArr){
System.out.println(exampleTweet);
}
Tweets.save(tweetArr);
Tweet[] reloadedTweets = Tweets.load();
for(Tweet tempTweets : reloadedTweets){
System.out.println(reloadedTweets);
}
}
}
package com.dfsweb;
import java.util.Date;
import java.io.Serializable;
public class Tweet implements Comparable, Serializable {
private String mAuthor;
private String mDescription;
private Date mCreationDate;
public Tweet (String author, String description, Date creationDate){
mAuthor = author;
mDescription = description;
mCreationDate = creationDate;
}
@Override
public String toString(){
return String.format("Tweet: \"%s\" by %s on %s",
mDescription, mAuthor, mCreationDate);
}
@Override
public int compareTo(Object obj){
Tweet other = (Tweet) obj;
if(equals(other)){
return 0;
}
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
if (dateCmp == 0){
return mDescription.compareTo(other.mDescription);
}
return dateCmp;
}
public String getAuthor(){
return mAuthor;
}
public String getDescription(){
return mDescription;
}
public Date getCreationDate(){
return mCreationDate;
}
public String[] getWords(){
return mDescription.toLowerCase().split("[^\\w#@'åäö]+");
}
}
package com.dfsweb;
import java.io.*;
public class Tweets {
public static void save(Tweet[] tweets){
try (
FileOutputStream fos = new FileOutputStream("tweets.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
) {
oos.writeObject(tweets);
} catch(IOException ioe) {
System.out.println("Problem saving");
ioe.printStackTrace();
}
}
public static Tweet[]load() {
Tweet[] tweets = new Tweet[0];
try(
FileInputStream fis = new FileInputStream("tweets.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
) {
tweets = (Tweet[]) ois.readObject();
} catch(IOException ioe) {
System.out.println("Problem loading");
ioe.printStackTrace();
} catch(ClassNotFoundException cnfe) {
System.out.println("Error loading tweets");
cnfe.printStackTrace();
}
return tweets;
}
}