Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial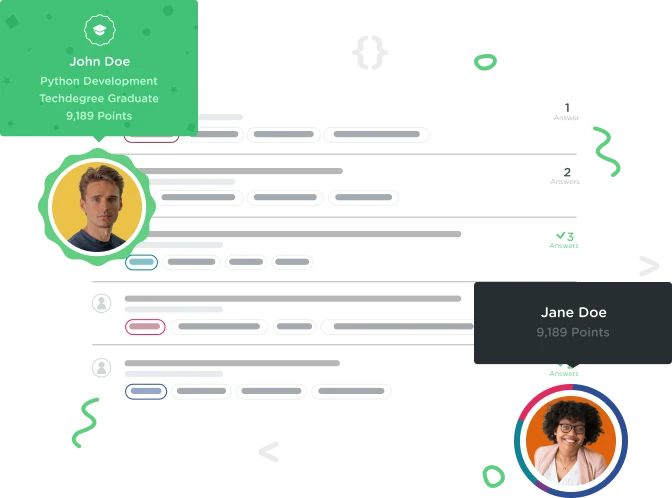
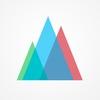
Chris Mason
Full Stack JavaScript Techdegree Graduate 24,526 PointsDeclaring a function with a parameter(s) or without, what's the difference?
If when declaring a function I don't put in a parameter, but intend to add an argument to the parameter space when calling the function, does it make any difference later to the behaviour of the code when the function is called (the fact no parameter was present in the function declaration but all of a sudden an argument has appeared)? or to put it another way, would there be any difference in performance between the two functions below, assuming I'm going to pass the same argument to each when called?
And also 3 other little related questions which I'd greatly appreciate some clarification on:
- does the choice of the parameter word have any significance?
- does the parameter word in the function declaration in any way computationally link to anything elsewhere in the JavaScript file or to the HTML or CSS in the programme files or serve in any way as some sort of coding instruction to the JavaScript engine?
- I see 'message' used a lot as the parameter word, is that just standard practice in the same way people tend to indent their code with two spaces or tab?
function printList( message ) {
var listHtml = '<ol>';
for ( var i = 0; i < placeholder.length; i += 1) {
listHtml += '<li>' + placeholder[i] + '</li>';
}
listHtml += '</ol>';
print(listHtml);
}
function printList( ) {
var listHtml = '<ol>';
for ( var i = 0; i < placeholder.length; i += 1) {
listHtml += '<li>' + placeholder[i] + '</li>';
}
listHtml += '</ol>';
print(listHtml);
}
Many thanks in advance!
4 Answers

Erik Nuber
20,629 PointsIf you call a function that has an argument expected, without an argument, it just sets it to undefined
function printList( message ) {
var listHtml = '<ol>';
for ( var i = 0; i < placeholder.length; i += 1) {
listHtml += '<li>' + placeholder[i] + '</li>';
}
listHtml += '</ol>';
print(listHtml);
}
printList();
Here message will be undefined. Which in this case matters little since it isn't actually used within the the function itself.
The only time it would matter is if that argument were important to the function
function doSomething(x) {
return (x * x + 3);
}
y = doSomething();
Here without X being passed in we would get NaN as x is undefined.
does the choice of the argument name have any significance?
No, though it would be best to use something that has some meaning you could use anything you like.
does the argument in the function declaration in any way computationally link to anything elsewhere in the JavaScript file or to the HTML or CSS in the program files or serve in any way as some sort of coding instruction to JavaScript engine?
This is a loaded question. As there are times when yes it does and times when no it doesn't. For where you are likely at in the courses the answer is going to be more often than not no. I would say yes though when you consider that functions can call functions, for recursive functions, for closure and for constructor functions. I am sure someone will weigh in on this.
You also can do things with that argument like pass it back out with a return statement so it really depends what you are asking. A function usually is meant to do something and, the arguments passed in are being passed in for a purpose. Either math, to create a statement, to find out a true/false statement etc...so it depends what the function is being used for.
var x = 3
function doSomething(x) {
x=4;
console.log(x); //gives 4
}
console.log(x) //gives 3
doSomething(x);
console.log(x)//gives 3
Notice here the difference, if I don't pass in an argument and change X which was globally created, it changes the x on a global scale. Above I created an argument called x so that is the only effected locally.
var x = 3
function doSomething() {
x=4;
console.log(x); //gives 4
}
console.log(x) //gives 3
doSomething();
console.log(x)//gives 4
I see 'message' used a lot as the parameter word, is that just standard practice in the same way people tend to indent their code with two spaces or tab?
Not sure about it being standard or best practice. I try to make my variables meaningful to what I am doing. Message is kind of versatile so not such a bad name to use.

Erik Nuber
20,629 Pointsfunction getArea (width, height) {
return width * height;
}
This is a more versatile function. You can pass in any width and height and get a result back. You can declare this over and over again with less lines of code.
var result = getArea(2, 5);
var result1 = getArea(5, 8);
var result2 = getArea(22, 35);
var result3 = getArea(4, 9);
You can achieve the same thing with...
function getArea () {
return width * height;
}
However, in this case if width and height are global, the above examples would take more lines of code and be less efficient.
width = 2;
height = 5;
var result = getArea();
width = 5;
height = 9;
var result1 = getArea();
width = 22;
height = 35;
var result2 = getArea();
width = 4;
height = 9;
var result3 = getArea();
for a few examples, it isn't that big of a deal but, if you had to do even fifty getArea calculations it would get very old resetting the width and height over and over again.
If you want to take it up a notch and add the function call multiple times it also may help solidify why the top function is more useful
result = getArea(2, 5) + getArea(5,9) + getArea(22, 35);
With this we are able to get several areas and add them together. If you had to do this with global variables only you would have to reset the variables several times and then add the results.
width = 2;
height = 5;
var result = getArea();
width = 5;
height = 9;
var result1 = getArea();
width = 22;
height = 35;
var result2 = getArea();
finalResult = result + result1 + result2
Both would lead to the same answer but, writing one line of code is so much easier and more efficient than 10 lines.
Hope that helps!
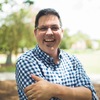
Dave McFarland
Treehouse TeacherHi Chris Mason
Erik Nuber's response is spot on, but I'll add my two cents as well. These two functions are essentially identical:
function printList( message ) {
var listHtml = '<ol>';
for ( var i = 0; i < placeholder.length; i += 1) {
listHtml += '<li>' + placeholder[i] + '</li>';
}
listHtml += '</ol>';
print(listHtml);
}
function printList( ) {
var listHtml = '<ol>';
for ( var i = 0; i < placeholder.length; i += 1) {
listHtml += '<li>' + placeholder[i] + '</li>';
}
listHtml += '</ol>';
print(listHtml);
}
Because you don't use the message
parameter in the first function, it isn't needed. A parameter is like a variable for the function -- when you call the function you "pass" an argument (that's a value like a string, number or even an array) to the function. The function stores that value in the "parameter" (the message
parameter in this example). Then the function can use that information to complete whatever the function is programmed to do. This is covered in depth in the section on "Functions" in the JavaScript Basics Course. In particular you can learn about parameters in the Giving Information to Functions video.
In your code example, you'd be better off passing in the value of the placeholder
value since you do use that within the function. This related to a concept called scope
which is a bit tricky, but an important part of understanding how JavaScript works. You can watch the Variable Scope video to learn more about that.
For your 3 questions:
- does the choice of the parameter word have any significance? No. It's a name you provide -- just like you do when you create variables. As I mentioned above, a parameter basically is just a variable for the function so you can name it whatever you like, but you should follow the same rules that you would when naming any variable. You can watch the Naming Variables video to learn more.
- does the parameter word in the function declaration in any way computationally link to anything elsewhere in the JavaScript file. Great question. No it does not. The parameter name is internal to the function -- in other words that name is just for the function's use and is not referenced anywhere else in the program. The video I pointed to above about variable scope should help make that clearer. This can get a bit confusing, because it's possible to use the same name OUTSIDE of the function and a parameter name INSIDE the function, but they remain separate "buckets" or variables. For example:
var userName = 'Dave';
function printName( userName ) {
console.log( userName );
}
// call the function and pass a value to it
printName('Chris'); // the word 'Chris' is printed to the JavaScript console in the browser
// log the userName (global variable)
console.log(userName); // the word 'Dave' is printed to the JavaScript console in the browser
This is probably really confusing to you right now -- but with more practice, seeing more code and taking more lessons here on Treehouse, you'll start to understand this.
-
I see 'message' used a lot as the parameter word, is that just standard practice? Not really. You can use any word you want (see the Naming Variables video I link to above). It's just that message is pretty descriptive for many cases. For example, if you created a function to open an alert dialogue with some text in it, the name
message
is pretty descriptive. But you could just as easily use a name likenote
,alertText
, or evenbob
(but that's not very descriptive, so I wouldn't usebob
as a variable name :)
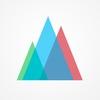
Chris Mason
Full Stack JavaScript Techdegree Graduate 24,526 PointsThank you Dave McFarland and ErikNuber both for the responses. Very helpful and I feel clearer on the issue. I can see now how the two quote examples I gave would be the same yes, as as you say ‘message’ isn’t used in either!
If I were to ask the question differently, (with an example where the parameter is actually used by the function!) would there be any difference between the two functions below? Assuming in both cases that width & height are two globally declared and accessible variables.
function getArea (width, height) {
return width * height;
}
function getArea () {
return width * height;
}
I guess I don’t really get why it’s necessary (obviously it is, and it’s a failure on my part) why one would need to put parameters into a function definition at all, when it seems the function can quite easily get the arguments that it needs later on when called (from within itself if the argument is a local variable or from the global space.
I take your point that this will probably all start to make more sense with time once the kinds of functions I’m working with are more complex and I become more used to working with them, so I’ll try not to get too hung up on the topic!