Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial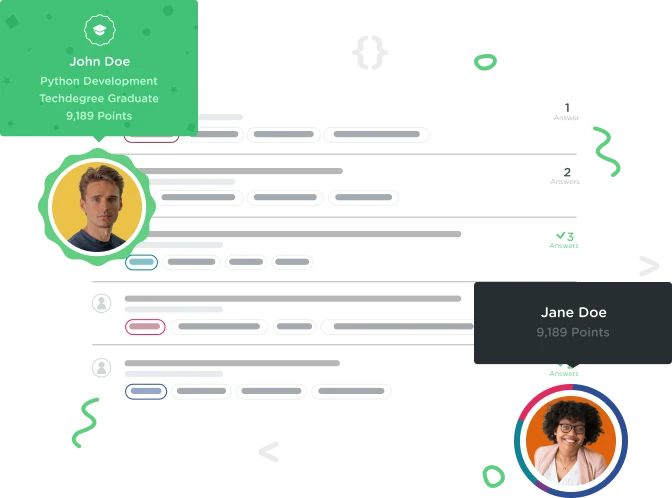
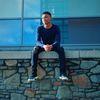
Nathan Marshall
6,031 PointsDeclaring a global variable in Jquery/Javascript
Hi Guys,
I'm currently creating an application which allows me to set a username and password for a user. For them to later repeat the same credentials and if they match an alert message pops up. I thought this would be a great way to practice my jquery skills.
I have decided to make my variables global and I was just wondering why are my global variables aren't being recognized when I refer to the inside of a function?
Is there something I am not understanding here?
If somebody could take their time to explain, that would be appreciated.
Here is my codepen - https://codepen.io/Nathan-Callum-Marshall/pen/VxMGjg?editors=1111
Thanks,
1 Answer

andren
28,558 PointsThe issue is when you assign values to the global variables. Variables are not dynamic, their value is static. As soon as you assign something to them that is the value they keep until you manually change them.
When the page loads your variables are declared and you initialize them with the current value of #set-username
and #set-password
:
var $username = $("#set-username").val();
var $password = $("#set-password").val();
Since the page has just loaded that value is just an empty string. When you reference these variables later in your code those elements might have a different value, but the variables have not had their value changed. So they still just contain an empty string.
If you split the declaration and initialization of your variables so that their value is assigned at the moment that you expect a username/password to have been entered like this:
var $username;
var $password;
$(".content-area").on("click","#saveDetails",function(event){
if( $("#set-username").val().length > 0 ) {
$username = $("#set-username").val();
console.log($username);
}
});
$(".content-area").on("click","#saveDetails",function(event){
if( $("#set-password").val().length > 0 ) {
$password = $("#set-password").val();
console.log($password);
}
});
Then your code will work. Though I have to say that attaching two separate click event listeners to #saveDetails
seems a bit wasteful, merging them into one listener like this:
var $username;
var $password;
$(".content-area").on("click","#saveDetails",function(event){
if( $("#set-username").val().length > 0 ) {
$username = $("#set-username").val();
console.log($username);
}
if( $("#set-password").val().length > 0 ) {
$password = $("#set-password").val();
console.log($password);
}
});
Is more efficient and will work just as well.
Nathan Marshall
6,031 PointsNathan Marshall
6,031 PointsThank you! Yeah, I thought that my code seemed long winded, I wasn't really sure what was the best way to lay it out. I'm still fairly new to this. Thanks for explaining it means a lot!