Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial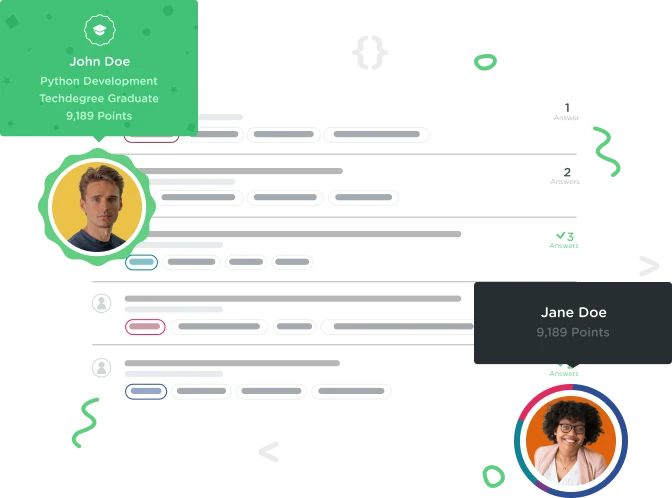

Asif Ahmed
2,881 PointsDeclaring a variable and initializing it to a different type
In this video, we declare items as a BlockingQueue but then initialize it as an ArrayBlockingQueue. What's happening here? When is this valid? How specific do we need to be when declaring a variable if we will later initialize it to a later object?
private BlockingQueue<Item> items;
public Bin(int maxItems) {
items = new ArrayBlockingQueue<>(maxItems);
}
1 Answer

jb30
44,807 PointsBlockingQueue is an interface. ArrayBlockingQueue is a class that implements BlockingQueue. In Java, we can't make an instance directly from an interface (BlockingQueue items = new BlockingQueue();
is not valid). We don't necessarily care which implementation of BlockingQueue we use, but we want to be able to call the methods that BlockingQueue promises will be implemented in anything that implements BlockingQueue.
Suppose you have a made-up class named SomeClass
which extends a made-up class SuperclassOfSomeClass
and implements a made-up interface InterfaceSomeClassImplements
.
We can use the same format when we have SuperclassOfSomeClass instanceName = new SomeClass();
or InterfaceSomeClassImplements instanceName = new SomeClass();
.
We do not need to be very specific when declaring a variable, but it is often a good idea to make your code as clear as you can. You could do Object instanceName = new SomeClass();
since all classes inherit from Object, but you would need to cast instanceName
to the class SomeClass
if you want to use methods from SomeClass
.