Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial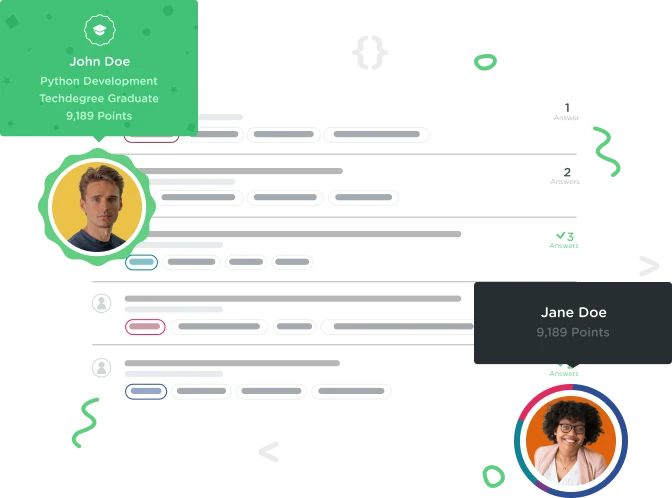

Kelly Walsh
3,642 PointsDeclaring Variables
Just curious - why can't you just declare the red, green, and blue variables at the top outside of the loop as listed below? I tried and it doesn't work, but I would like to know why. Thank you!
var html = ''; var red = Math.floor(Math.random() * 256 ); var green = Math.floor(Math.random() * 256 ); var blue = Math.floor(Math.random() * 256 );
5 Answers
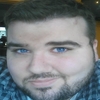
Marcus Parsons
15,719 PointsHi Kelly,
There's no reason why it shouldn't work to declare the variables outside of the function. It's generally not a good idea to keep variables in the global scope like that, though, because common names of variables tend to get reused often in other scripts and may cause a conflict. It's best to go ahead and get in the habit of declaring local variables and using them that way.
But, with that being said, here's how you can use red, green, and blue outside of a loop with the refactoring challenge:
//create them outside of the loop
var html = '', red, green, blue, rgbColor;
for (var i = 0; i < 10; i += 1){
//Get red green and blue colors
//set them inside the loop
//do not use var keyword again when setting variables
red = Math.floor(Math.random() * 256);
green = Math.floor(Math.random() * 256);
blue = Math.floor(Math.random() * 256);
//Compile the colors into a CSS rgb format
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
//Initialize each div to be perfect circles width and height are equal and border-radius 100%
//Set background color to the rgb received
html += '<div style="border-radius:100%;width:50px;height:50px;background-color:'+rgbColor+'"></div>';
}
//When all divs are created, write the html to the screen
document.write(html);

Kelly Walsh
3,642 PointsThank you!
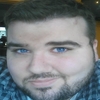
Marcus Parsons
15,719 PointsYou're very welcome, Kelly! Do you have any more questions?

Kelly Walsh
3,642 PointsNot yet! I'm sure I'll have more in time.
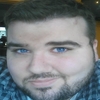
Marcus Parsons
15,719 PointsGood deal! Happy Coding, Kelly =]

Michal Jankowski
4,051 PointsHello Marcus,
I saw your code and I think it's really cool!
I didn't know that it's possible to declare the variables like you did (all at once): // var html = '', red, green, blue, rgbColor;
It means that we have one variable with many values?
Could you give me a hint how should I understand it?
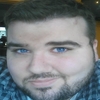
Marcus Parsons
15,719 PointsHi Michal,
What you are seeing there is a shorthand way to initialize multiple variables on one line. All you have to do is separate each variable/value pair with a comma. If you don't have to initialize the variable with a default value, then you can just write the variable name and a comma.
So, here is the code so you can see it:
//this
var html = '', red, green, blue, rgbColor;
//is the same as:
var html = '';
var red;
var green;
var blue;
var rgbColor;

Michal Jankowski
4,051 PointsThank you Marcus, it's clear now.
I saw your website and your aside menu effect is really nice ;)
Keep going, because you're doing great!
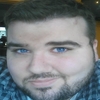
Marcus Parsons
15,719 PointsYou're welcome and thank you, Michal! I appreciate the compliment on my website :) I've poured some hours into it haha It was written completely from scratch by me. You keep going, too, and I'm sure you're gonna do awesome things! Happy Coding, Michal!

Michal Jankowski
4,051 Pointshaha practice makes perfect! ;)
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsI forgot to mention that I did a variation of this challenge for the randomness part of my portfolio: http://marcusparsons.com/projects/randomcolorcircles/index.html