Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial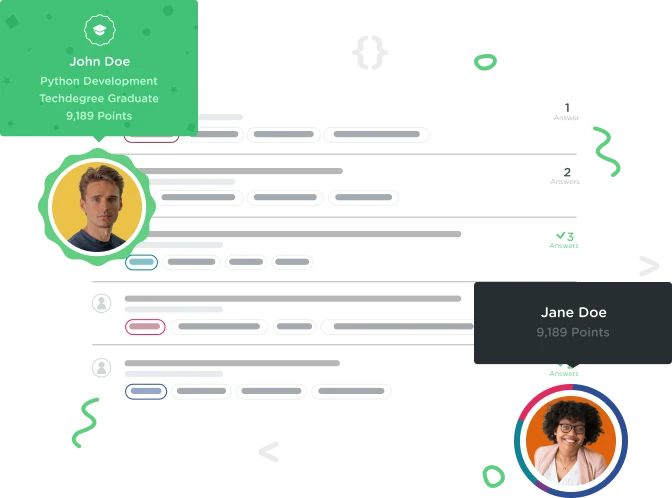
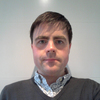
James Pask
13,600 PointsDecreasing the mBarsCount whilst completing a lap on the code challenge
I'm attempting the coding challenge to decrease the mBarsCount by 1 when driving a lap around the track. I have the code below but get this error when "checking":
Bummer! Remember that you should call the existing drive method and pass in the default parameter of 1. Otherwise you would have to rewrite all that (omitted) code.
How do I call the existing drive method and pass in the default value of 1?
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void drive() {
int laps = 1;
mBarsCount--;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers
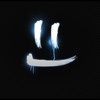
Grigorij Schleifer
10,365 PointsHi James,
You can create a method with the same name, but with an another method signature. So you get two methods with different signatures.
//signature 1 (int lap)
public void drive(int lap){
vs.
//no arguments
public void drive(){
In the new method call the drive method and pass one lap inside.
public void drive(){
drive(1);
}
drive(1);
This part of code calls the first drive method and this method takes an int 1 = one lap (like the signature of this method).
Grigorij
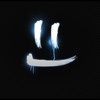
Grigorij Schleifer
10,365 PointsGood morning,
You can call the isFullyCharged() method. Then your mBarsCount will be set up to MAX_BARS. So the mBarsCount will be 8. If you call the drive() method with 3 laps .... your mBarsCount will be decremented 3 times to mBarsCount = 5.
James Pask
13,600 PointsJames Pask
13,600 PointsSo:
drive(1);
does something similar to what I had of
int laps = 1;
What I had seemed to work and not give me any compile errors.
Does the "default" = 1 because we've set "drive(int lap)" - i.e. lap = 1 lap
If we were to drive round the track, say 3 times, would we simply change the value to 3:
Hopefully I'll soon get my head around this!