Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial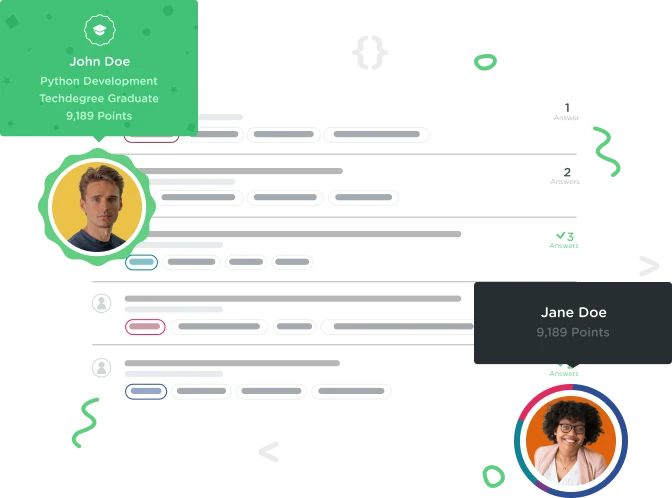

Igor Bochenek
3,960 Pointsdef covers_all
Create a new function named covers_all that takes a single set as an argument. Return the names of all of the courses, in a list, where all of the topics in the supplied set are covered. For example, covers_all({"conditions", "input"}) would return ["Python Basics", "Ruby Basics"]. Java Basics and PHP Basics would be exclude because they don't include both of those topics. I'm stuck in second step
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(setss):
lists = []
for key, value in COURSES.items():
if value & setss:
lists.append(key)
return lists
covers({'Ruby'})
def covers_all(sett):
listt = []
for key, value in COURSES.items():
if <= sett:
return listt
covers({"conditions", "input"})
9 Answers
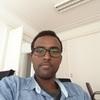
Mubarig CISMAN
Python Web Development Techdegree Student 2,547 PointsOne needs to check if both sets have all the same values, the supplied SET and the COURSES SET. This has worked for me .
def covers_all(topics):
courses_list = []
for course, values in COURSES.items():
if(values & topics) == topics:
courses_list.append(course)
return courses_list
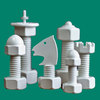
Steven Parker
230,688 PointsTask 2 is only slightly different from task 1
In task 1 you checked to see if the intersection of the sets was not empty.
For task 2, what if you did the same thing, but tested if the intersection of the sets was the same as argument set itself?
I'll bet you can get it now without a code spoiler.

Vitaly Pankratov
2,641 PointsThank you so much!
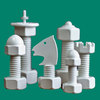
Steven Parker
230,688 PointsChris, if you apply the hint that I gave Igor 2 months ago, your test might look something like this:
if topics & COURSES[course] == topics: # if covered topics is the same as all topics
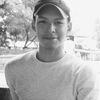
edwardperez
Full Stack JavaScript Techdegree Graduate 23,796 PointsIn the previous video towards the end, he mentions a few methods you should check out from the link in the teacher's notes. One being the issubset() method (keyword sub) which returns True if all items in the set exists in the specified set, otherwise it returns False. There is a issuperset() method as well which will return False in this case so please do reference the provided links to understand the difference and how to implement. I am just starting like everyone else but this code is much cleaner and passes the test. Good luck, everyone! Update ==> Please do check Steven Parker comment below for a more concise if statement using math operators!
def covers_all(arg):
output = []
for title, course in COURSES.items():
#if arg is within the course return True and append title to output
if arg.issubset(course):
output.append(title)
return(output)
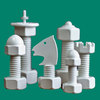
Steven Parker
230,688 PointsThere's also a set operator to perform this same check with even more concisely:
if arg <= course:
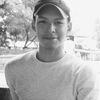
edwardperez
Full Stack JavaScript Techdegree Graduate 23,796 PointsThat's so clean and simple Steven Parker! I did not realize you could use simple math operators, learn something new! Thank you and I will definitely save your code in my practice file!
Chris Grazioli
31,225 PointsNot sure why this doesn't work, should I be using subset or something like it. I thought I was just testing to see if the topics where in the set COURSES[course].
def covers(topics):
list_of_courses=[]
for course in COURSES.keys():
if topics & COURSES[course]:
list_of_courses.append(course)
return list_of_courses
def covers_all(topics):
list_of_courses=[]
for course in COURSES.keys():
if topics in COURSES[course]:
list_of_courses.append(course)
return list_of_courses
I'd be pulling my hair out over this one if I wasn't already bald!

Dê Gìa
iOS Development Techdegree Student 26,430 PointsHave you got the answer to this question? I have the same solution and now I get stuck and don't know what's is wrong. Thanks ...

Yougang Sun
Courses Plus Student 2,086 Pointsif topics in COURSES[course]... the 'set' topics can not be 'in' the 'list' COURSE[course]. You can write: if (topics & set(COURSES[course])) == topics: list_of_courses.append(course)
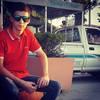
Robert Stepanyan
2,297 PointsI'd finally got it if you don't get the 2nd task but understood how to solve the first one then you can understand this one too So, at the first task, you only check if the intersection contains anything because there is only one argument to check that means that if the intersection is not empty function returns a key. For the second task, you also need to check if there two arguments in intersection (if value.intersection(arguments) == arguments)
def covers_all(arguments):
outputs = []
for key, value in COURSES.items():
if value.intersection(arguments) == arguments:
outputs.append(key)
return outputs

Vashisth Bhatt
Python Web Development Techdegree Student 6,786 Pointsif value.intersection(arguments) == arguments: outputs.append(key)
Can you explain in detail what does this line is exactly doing.It will be great help

Vashisth Bhatt
Python Web Development Techdegree Student 6,786 Pointsif value.intersection(arguments) == arguments: outputs.append(key)
Can somebody explain in detail what does this line is exactly doing.It will be great help

Viktor Worldchanger
9,765 Pointsdef covers_all(arg):
courses_list = []
for course, topics in COURSES.items():
if arg - topics == set():
courses_list.append(course)
return courses_list
I found that if there is no output for difference(meaning that a set is fully included in another set) we have set() as output
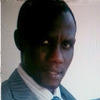
BENJAMIN UWIMANA
Courses Plus Student 22,636 PointsCOURSES = { "Python Basics": {"Python", "functions", "variables", "booleans", "integers", "floats", "arrays", "strings", "exceptions", "conditions", "input", "loops"}, "Java Basics": {"Java", "strings", "variables", "input", "exceptions", "integers", "booleans", "loops"}, "PHP Basics": {"PHP", "variables", "conditions", "integers", "floats", "strings", "booleans", "HTML"}, "Ruby Basics": {"Ruby", "strings", "floats", "integers", "conditions", "functions", "input"} }
def covers(topics_set): overlap_list = [] for topics, courses in COURSES.items(): for topic in topics_set: if topic in topics_set.intersection(courses): overlap_list.append(topics) return overlap_list
def covers_all(topics_set): overlap_list=[] for topics,courses in COURSES.items(): #if topics_set.intersection(courses) == topics_set: if topics_set.issubset(courses): overlap_list.append(topics) return overlap_list
print(covers({"Python", "Java"})) print(covers_all({"conditions", "input"}))
Jebadeiah Jones
10,429 PointsJebadeiah Jones
10,429 PointsOh my goodness...
I had gone through the whole process and written this up, trying everything I could imagine to make my code work. It was driving me absolutely mad, so I finally decided I would look up the answer and see where I'd gone wrong.
'==', not '='.
Aaaaaaaaaagh!