Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial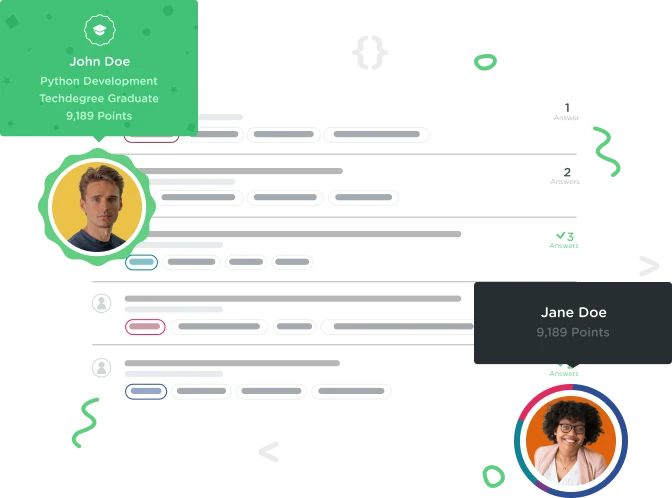

Andrew Bickham
1,461 Pointsdef move
I put out one of these earlier but this is an edited version, I know im still missing in some area, please advise
# EXAMPLES:
# move((1, 1, 10), (-1, 0)) => (0, 1, 10)
# move((0, 1, 10), (-1, 0)) => (0, 1, 5)
# move((0, 9, 5), (0, 1)) => (0, 9, 0)
def move(player,direction):
x, y, hp = player
d1, d2 = direction
moves = ["Left", "Right", "Up", "Down"]
if x + d1 == 0:
moves.remove("Left")
elif x == 0 + d1 == 0 - 1:
hp -= 5
if x == 9:
moves.remove("Right")
elif x + d1 == 9 + 1:
hp -= 5
if y + d2 == 0:
moves.remove("UP")
elif y + d2 == 0 -1:
hp -= 5
if y + d2 == 9:
moves.remove("Down")
elif y + d2 == 9 + 1:
hp -= 5
return x,y,hp
3 Answers

Andrew Bickham
1,461 Pointshey josh, I hear what your saying the only thing im not 110% sure on in your coding is that after each "if" & "elif" statements you'll have a bit of code that reads x = 0 and x = 9, and then also y = 0 and y = 9. I get everything else but those few bits.

Josh Keenan
20,315 PointsHere's my solution to this:
def move(player, direction):
x, y, hp = player
x += direction[0]
y += direction[1]
if x > 9:
x = 9
hp -= 5
elif x < 0:
x = 0
hp -= 5
if y > 9:
y = 9
hp -= 5
elif y < 0:
y = 0
hp -=5
return x, y, hp
So all that needs to be done is to check if the player is leaving the permitted area. To do this we need to take the new movements and add them to the current position, if they are negative they will go down, if it is positive it will be added fine.
After that we need to check if the player is still on the board, if not we need to put them back on it, they will be off the board if one of four conditions are met, the x or y is above 9, or the x or y is below 0. So we test for them, but since it can't be both greater and less than the limit on any one axis, we can use and if/elif clause without an else, as we don't need to do anything in the situation that none of these conditions are met.
Hope this makes sense and helps, feel free to ask any questions!

Josh Keenan
20,315 PointsAlso, we know that the direction will be packed as an x, y so direction[0] will be the x and direction[1] the y

Andrew Bickham
1,461 Pointsok i gotcha thank you for the help and simplifying everything I really do appreciate it

Josh Keenan
20,315 PointsAnytime mate, all the best, you got this just keep going!
Josh Keenan
20,315 PointsJosh Keenan
20,315 Pointsso you want to keep the player on the board, the board is going from 0 to 9 in each direction. The if/elif is testing to see if the player is outside of those bounds, if the player is over 9, they have hit the wall, so we move them back onto the board at either 9 or 0, so we have to set their x or y position to the correct one. We also remove some hp as well as they have just ran into the wall.
I coded it this way as it is simpler to do the math and move the player, then check if they are still within the confines of the board, it is easier to write this if/elif twice and not code for however many other potential scenarios there would be if we tried to do the checking another way. Hope this makes sense