Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial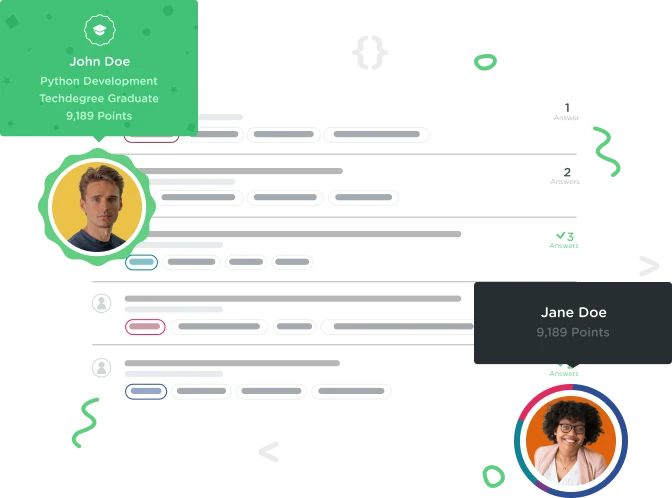
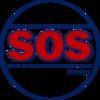
SOS Norway
4,219 Pointsdef run method error msg
Tors-MacBook-Pro:Ruby cherrybluemoon$ ruby address_book.rb
address_book.rb:10:in `<class:AddressBook>': undefined local variable or method `address_book' for AddressBook:Class (NameError)
from address_book.rb:3:in `<main>'
require "./contact"
class AddressBook
attr_reader :contacts
def initialize
@contacts = []
end
Β Β def run
loop do
puts "Address Book"
puts "a: Add Contact"
puts "p: Print Address Book"
puts "e: Exit"
print 'Enter your choice: '
input = gets.chomp
case input
when 'e'
break
end
end
end
def print_search(search, results)
puts search
results.each do |contact|
puts contact.to_s('full_name')
contact.print_phone_numbers
contact.print_addresses
puts "\n"
end
end
def find_by_name(name)
results = []
search = name.downcase
contacts.each do |contact|
if contact.full_name.downcase.include?(search)
results.push(contact)
end
end
print_search("Name search results (#{search})", results)
end
def find_by_phone_number(number)
results = []
search = number.gsub("-", "")
contacts.each do |contact|
contact.phone_numbers.each do |phone_number|
if phone_number.number.gsub("-", "").include?(search)
results.push(contact) unless results.include?(contact)
end
end
end
print_search("Phone search results (#{search})", results)
end
def find_by_address(address)
results = []
search = address.downcase
contacts.each do |contact|
contact.addresses.each do |address|
if address.to_s('long').downcase.include?(search)
results.push(contact) unless results.include?(contact)
end
end
end
print_search("Address search results (#{search})", results)
end
address_book = AddressBook.new
address_book.run
2 Answers
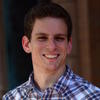
Jeff Lange
8,788 PointsYou're missing a closing end
on your run
method
def run
loop do
puts "Address Book"
puts "a: Add Contact"
puts "p: Print Address Book"
puts "e: Exit"
print 'Enter your choice: '
input = gets.chomp
case input
when 'e'
break
end # <= you're missing this end
end
end
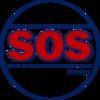
SOS Norway
4,219 PointsThanks Jeff!
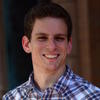
Jeff Lange
8,788 PointsYou're welcome! Keep your indents clean and it will help you spot them. :)