Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial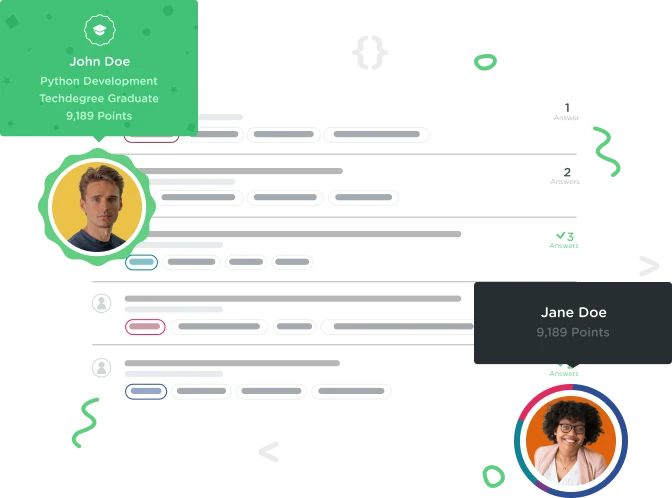

Andrew Bickham
1,461 Pointsdef word_count
I keep getting a type error that say "must be str, not list" which i am confused about, if someone could hint at what im doing wrong that would be greatly appreciated
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(sentence):
words = sentence.lower().split()
for word in words:
result = dict(word.count(words))
return result
2 Answers
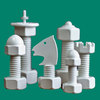
Steven Parker
230,274 PointsI believe the message is complaining about the string version of "count" being passed a list argument instead of a substring. But even if that is fixed, "count" will return a single int value, which by itself would not be sufficient data to create a new dictionary from.
And a couple more hints:
- you'll want to build up a dictionary instead of creating a new one for each word
- the "return" should be moved out of the loop to avoid ending it prematurely on the first pass

Andrew Bickham
1,461 Pointslol as simple as that thank you!!
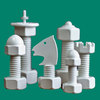
Steven Parker
230,274 PointsAndrew Bickham — Glad to help. You can mark a question solved by choosing a "best answer".
And happy coding!
Andrew Bickham
1,461 PointsAndrew Bickham
1,461 Pointsokay so i understood the hint where you said i needed to build a dictionary so i added an empty one and build it that way, so if you could give me one or two more pointers, to help crack this code, everytime i try and clear the challenge all it says is "try again"
Andrew Bickham
1,461 PointsAndrew Bickham
1,461 PointsI made some correction but i still keep getting a bummer message that says, try again, if you hint at what the problem is that would be greatly appreciated it
Steven Parker
230,274 PointsSteven Parker
230,274 PointsYou've essentially got it now.
The only remaining issue is simply formatting — the "return" statement is indented just a bit too much to line up with the other items at the same indentation level.
Fix that and you'll pass.