Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial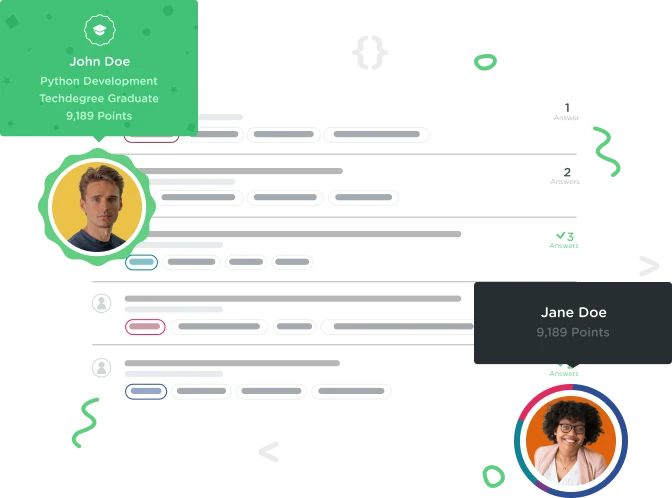

Akos Turi
7,517 PointsDefault will never be executed
enum Day {
case Monday
case Tuesday
case Wednesday
case Thursday
case Friday
case Saturday
case Sunday
}
func weekdayOrWeekend(dayOfWeek: Day) -> String {
switch dayOfWeek {
case Day.Monday, Day.Tuesday, Day.Wednesday, Day.Thursday, Day.Friday:
return "It's a weekday"
case Day.Saturday, Day.Sunday:
return "It's the weekend"
default:
return "Not a valid day"
}
}
weekdayOrWeekend(Day.Sunday)
the code itself works fine but when i try to give an invalid value, it says default can't be executed and get an error
5 Answers
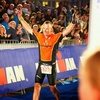
Steve Hunter
57,712 PointsHi Akos,
The code does work fine. What error value are you trying to submit?
If you send the method something that isn't a valid Day
value, the method call will error rather than the default
value being used. If you use a valid Day
value, then the switch will pick that up accordingly.
I get a warning that the default
will never be used, and that's correct; you have created a mutually exclusive set of rules; you can't submit an error into the switch; that will fail at the method call.
var errorValue: Int
weekdayOrWeekend("Steve") // Cannot convert value of type String to expected argument type Day
weekdayOrWeekend(errorValue) // cannot convert Int to Day
What behaviour were you expecting from this; what's not acting like you wanted it to?
Steve.

Akos Turi
7,517 Pointsi got an warning that default will never be executed, so probably that is your second thought, what you have mentioned. I just do not understand why that code works for Amit perfectly but not for me, why do i get a warning. Thanks for your help in advance
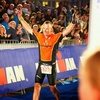
Steve Hunter
57,712 PointsI would say that this is a difference between Swift v1 and Swift v2.x which you are running. The later version tightened up on the typing aspects of Swift so there are some real differences between the code Amit is using and yours. Either way, your code works fine; indeed, it is more logical not requiring the default
as there cannot be any use for it; if you get beyond the method call, you've done enough testing of the parameter so no default is required.
Steve.
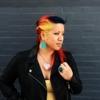
Dar Phan
1,459 PointsHey Steve,
I'm running the most recent version and I'm having the error, which I figured was fine. However, when I call a different day my output doesn't change.
For example, I call Friday, it tells me it's a weekday and it shows two warning messages. Then I change it to Saturday, it still says weekday and same warning messages. I change it to none of the days above, output has an error. Why am I changing it from weekend to weekday but the output isn't changing?
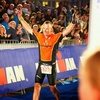
Steve Hunter
57,712 PointsCan you post your code and I'll take a look.
Thanks,
Steve.
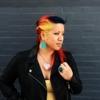
Dar Phan
1,459 Pointsenum Day {
case Monday
case Tuesday
case Wednesday
case Thursday
case Friday
case Saturday
case Sunday
}
let days = ["Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"]
func weekedayOrWeekend(dayOfWeek: Day) -> String {
switch dayOfWeek {
case Day.Monday, Day.Tuesday, Day.Wednesday, Day.Thursday, Day.Friday, Day.Saturday, Day.Sunday:
return "It's a Weekday."
case Day.Saturday, Day.Sunday:
return "Fuck yeah!"
default:
return "Not a Valid Day."
}
}
weekedayOrWeekend (Day.Sunday)
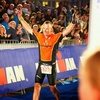
Steve Hunter
57,712 PointsHi Dar,
You have included Saturday and Sunday in your "It's a weekday" switch option so every day of the week will reach that line of code first, outputting "It's a weekday!". Remove Day.Saturday
and Day.Sunday
from that switch test and the code will then reach the second option.
Let me know how you get on.
Steve.

Akos Turi
7,517 PointsAll hail to Mr. Hunter!!! thanks for your effort
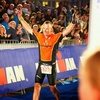
Steve Hunter
57,712 PointsHaha! Thank you. :-)
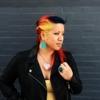
Dar Phan
1,459 PointsDo'h! Sometimes you just need another set of eyes. Thanks!