Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial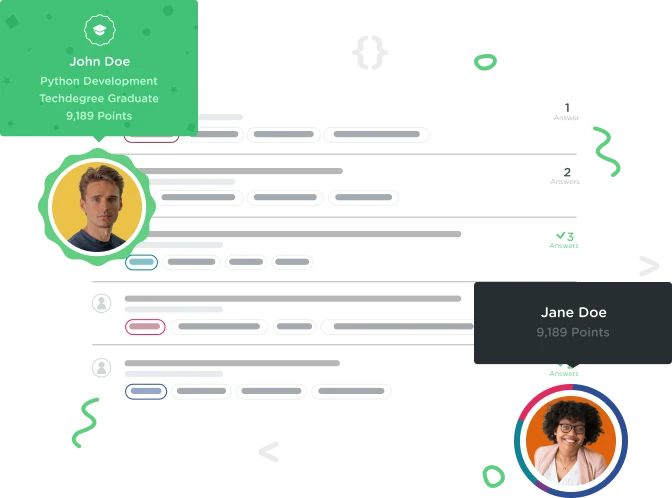

Ruth O'Kelly
4,944 PointsDeffered Execution?
At 1:52, James says "let's look at an example of deferred execution". Where was the example? I felt it wasn't clear exactly what was the example of this in the code he then wrote.
1 Answer

slaguerta
9,833 PointsIf you go to the 4 minute mark of the List Queries video, you'll find a good definition and example of deferred execution for a review. With deferred execution, a query won't be executed against the database until it's enumerated, either by a loop or calling a method like ToList() or Count.
So here's the code from this video, and I've put in some comments on where I think the query is finally being executed (before the execution, the LINQ query is defining the data you'll want back, but doesn't actually query the database yet until the data is requested).
//deferred execution example
var comicBooksQuery = context.ComicBooks
.Include(cb => cb.Series)
.OrderByDescending(cb => cb.IssueNumber); //not executed, sort of defining the data set in a query here
var comicBooks = comicBooksQuery.ToList(); //query is executed because of ToList method
var comicBooks2 = comicBooksQuery //query begins to be executed here because of the ToList Method
.Where(cb => cb.AverageRating < 7)
.ToList();
foreach(var comicBook in comicBooks) //query is executed because enumerating over a loop
{
Console.WriteLine(comicBook.DisplayText);
}
Console.WriteLine();
Console.WriteLine("# of comic books {0}", comicBooks.Count); //query sent here
Console.WriteLine();
foreach(var comicBook in comicBooks2) //query sent here
{
Console.WriteLine(comicBook.DisplayText);
}
Console.WriteLine();
Console.WriteLine("# of comic books {0}", comicBooks2.Count); //query sent here
I also found this article to be extremely helpful in kind of detailing deferred execution. (https://blogs.msdn.microsoft.com/charlie/2007/12/10/linq-and-deferred-execution/)
I would also step through the code with the debugger and breakpoints to kind of see how it is working like we do at the end of the "List Queries" video.
Simon Coates
28,694 PointsSimon Coates
28,694 PointsI'm struggling with that detail myself. C# is torturing me with magical or only partially explained advanced features, resulting in a perpetual nagging fear that something could go wrong at any second and i'll be left surveying smoking wreckage (and i had such a sunny disposition). Currently, i'm thinking of a query as just the idea of a query with execution of it happening invisibly at moments when you need a value (iteration, toList, Count, etc). But that seems too simple, so I'd assume there's some nuance I'm missing. FYI, getting responses on C# courses (especially advanced ones) can be a little tricky. You can always try tagging James Churchill to see if you get a response.