Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial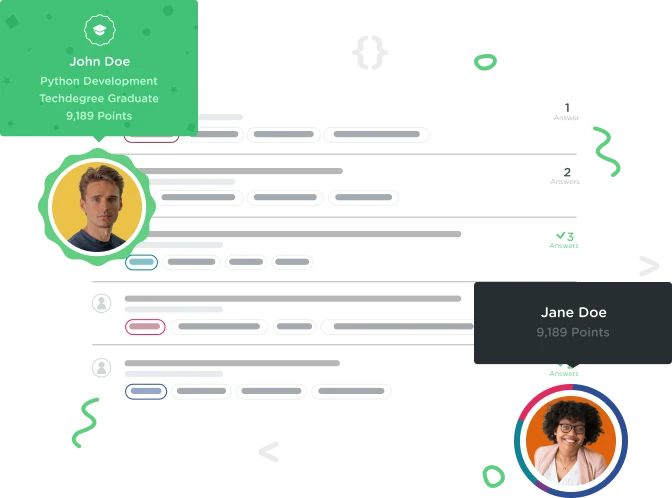

Jenny P
829 PointsDefine a Multiply function that accepts two parameters
So how am I suppose to do this? I get the right result in the console.
using System;
class Program
{
static double Multiply(double a, double b){
return a*b;
}
// YOUR CODE HERE: Define a method named Multiply. Remember
// to use the "static" and "void" keywords: "static void
// Multiply" (without quotes). Multiply should take two
// "double" values as parameters.
static void Main(string[] args)
{
Console.WriteLine(Multiply(2, 2.5));
Console.WriteLine(Multiply(6,7));
// YOUR CODE HERE:
// Call Multiply with two arguments: 2.5 and 2.
// YOUR CODE HERE:
// Call Multiply with two arguments: 6 and 7.
}
}
2 Answers
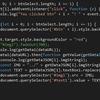
Damien Bactawar
8,549 PointsIn the question it instructs you to use the key words "static" and "void". You didn't use "void" and are returning a value as a "double". Void methods do not return anything, they just execute whatever lines are inside them.
Replace "static double Multiply..." with "static void Multiply..." and type "Console.WriteLine(a*b);" instead of "reutrn a*b". Also in "Main" just call the methods like "Multiply(2, 2.5)".
I hope this helps!

Jenny P
829 PointsThanks! I miss understood the static void thing and what it means to just "call" and not "return". Thanks for making it clear.