Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial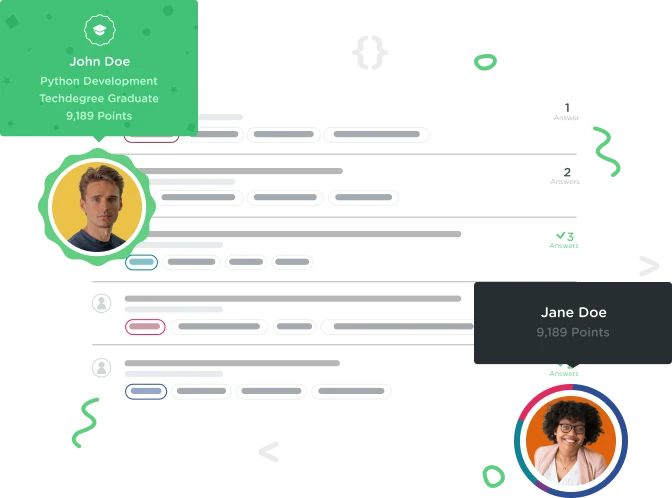

Tom Hartley
311 PointsDefine a Quote method that accepts a string parameter. It should return that same string, but surrounded by double quote
Define a Quote method that accepts a string parameter. It should return that same string, surrounded by double quotes.
using System;
class Program
{
// YOUR CODE HERE: Define a Quote method!
static string Quote(string phrase)
{
return Quote($"{phrase}");
}
static void Main(string[] args)
{
// Quote by Maya Angelou.
Console.WriteLine(Quote("When you learn, teach. When you get, give."));
// Quote by Benjamin Franklin.
Console.WriteLine(Quote("No gains without pains."));
}
}
2 Answers
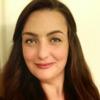
Jennifer Nordell
Treehouse TeacherHi there, Tom Hartley ! I received your request for assistance. First, you are attempting to call the Quote
method from within itself and this will cause an error without referencing the class first. But you don't need recursion here. The key lies in that we want the phrase to show the quotes as part of the string. To do this, you will need to escape the quotation marks. Generally, a quote mark means to C# to start a string, and end a string. But in this case, we want quote marks as part of the string.
static string Quote(string phrase)
{
// note that the quotes inside the string will have to be escaped
// so that they aren't considered the start of the string nor the end
return $"\"{phrase}\"";
}
Make sure you look at the preview of this code so that you understand the output. Hope this helps!

Dan Egan
1,118 PointsThank you fr writing this. It had me confused for days.