Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial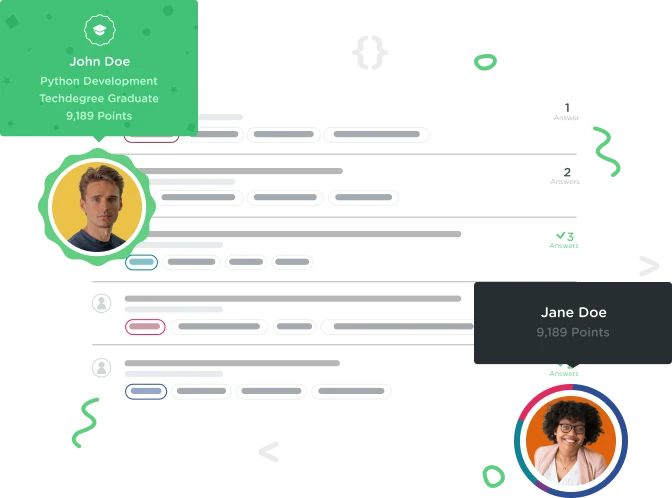
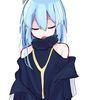
Kurobe Kuro^T_T^
5,369 PointsDefine an Eat method that takes two string parameters. Eat should return a string in the form "I think [first parameter]
i dong get it
using System;
class Program
{
// YOUR CODE HERE: Define an Eat method!
static void Eat(string have)
{
}
static void Main(string[] args)
{
Console.WriteLine(Eat("apples", "blueberries"));
Console.WriteLine(Eat("carrots", "daikon"));
}
}
4 Answers

Tammi Thomas
5,250 Pointsusing System;
class Program
{
static string Eat(string first, string second)
{
Console.Write($"I think {first} and {second} are tasty!");
return Console.ReadLine();
}
static void Main()
{
Console.WriteLine(Eat("apples", "blueberries"));
Console.WriteLine(Eat("carrots", "daikon"));
}
}
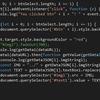
Damien Bactawar
8,549 Pointsstatic string Eat(string first, string second)
{
string result = "I think " + first + " and " + second +" are tasty!";
return result;
}
static string Eat(string first, string second)
{
return $"I think {first} and {second} are tasty!";
}
Both of the methods pass the challenge. The first method is using string concatenation and the second method is string interpolation.
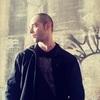
David Franco
3,252 PointsSpoiler Alert:
using System;
class Program {
static string Eat(string first, string second) { Console.WriteLine($"I think " + first + " and " + second + " are tasty!");
return $"I think {first} and {second} are tasty!";
}
static void Main(string[] args) { Console.WriteLine(Eat("apples", "blueberries")); Console.WriteLine(Eat("carrots", "daikon")); }
}

Stephen Stewart
5,727 PointsYou can get rid of the first console.writeline in teh Eat method as it is just duplicate code that shows up, and just have the return.

Jack Ernsberger
2,479 Pointswhat