Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial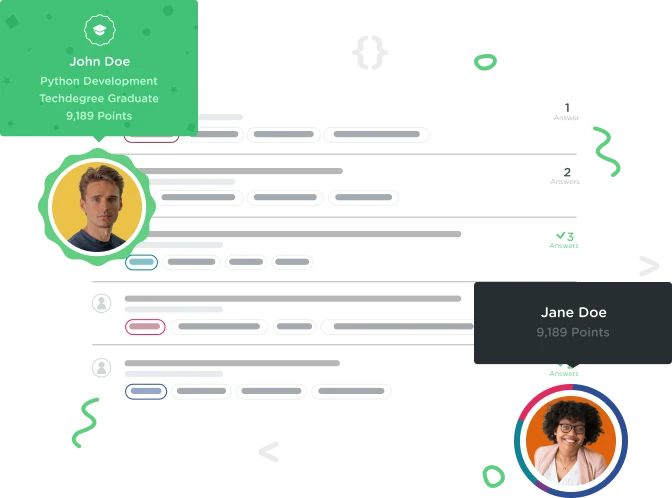
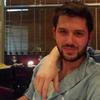
Nir Frank
3,578 PointsDefining variable inside loop works, defining outside of loop doesnt?
This works:
for (var x = 1; x < 101; x = x + 1) {
var divisibleByThree = x % 3;
var divisbleByFive = x % 5;
if (divisibleByThree === 0 && divisbleByFive === 0) {
console.log("fizzbuzz");
}
else if (divisibleByThree === 0) {
console.log("fizz");
}
else if (divisbleByFive === 0) {
console.log("buzz");
}
else {
console.log(x);
}
}
But this doesn't, and just counts from 1 to 100:
var divisibleByThree = x % 3;
var divisbleByFive = x % 5;
for (var x = 1; x < 101; x = x + 1) {
if (divisibleByThree === 0 && divisbleByFive === 0) {
console.log("fizzbuzz");
}
else if (divisibleByThree === 0) {
console.log("fizz");
}
else if (divisbleByFive === 0) {
console.log("buzz");
}
else {
console.log(x);
}
}
How come?
3 Answers
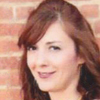
Alessandra Vaughn
13,915 PointsHi there,
When you are defining the variable outside of the loop x has no value. Once it is inside the loop it has a value.

Andrew Lima
5,233 PointsHi,
As Alessandra mentioned X has no value as it is out of scope.
You can access a variable from a higher scope (i.e. global variable within a function but you can't access a variable within a function globally)
Simply add this line of code under your global variables
var x = 1;
You can remove this section from your if statement :)
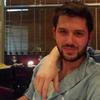
Nir Frank
3,578 PointsHey Andrew, thank you for your answer! It brought up another question I had, using a while () loop instead of a for () loop:
This works:
var x = 0;
var divisibleByThree;
var divisibleByFive;
while (x < 100) {
x = x + 1;
divisibleByThree = x % 3;
divisibleByFive = x % 5;
if (divisibleByThree === 0 && divisibleByFive === 0) {
console.log("fizzbuzz");
}
else if (divisibleByThree === 0) {
console.log("fizz");
}
else if (divisibleByFive === 0) {
console.log("buzz");
}
else {
console.log(x);
}
}
While this does not, and just gives me 100 instances of "fizzbuzz":
var x = 0;
var divisibleByThree = x % 3;
var divisibleByFive = x % 5;
while (x < 100) {
x = x + 1;
if (divisibleByThree === 0 && divisibleByFive === 0) {
console.log("fizzbuzz");
}
else if (divisibleByThree === 0) {
console.log("fizz");
}
else if (divisibleByFive === 0) {
console.log("buzz");
}
else {
console.log(x);
}
}
Also, I thought of placing the "var x = 0" after the divisibleBy variables - this just counts from 1 - 100.
What sort of "logic" (not in the programming sense, in the layman's terms sense) am I missing here?
If we're able to define variables out of scope, and then call on them within our loop, how come this doesn't work?
(Also, what do you mean I can remove the var x = 1; from my if statement? My (obviously wrong :P) understanding is that I'm defining the variable inside of the for (q; w; e) loop (putting it in place of the "q"), not inside of the if statement. Is that by chance what you meant? If so, how would I enter a "blank" value for "q"?)
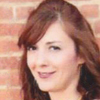
Alessandra Vaughn
13,915 PointsThe divisibleByThree and divisibleByFive variables are being set once, since they are outside of the loop. They need to be inside the loop so that they get set 100 different times and get to use the x that changes on each loop. It's basically the same problem as last time.
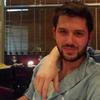
Nir Frank
3,578 PointsAwesome. Got it! Thanks Alessandra!!
Nir Frank
3,578 PointsNir Frank
3,578 PointsHey Alessandra, thanks for your answer! It brought up another question I had, using a while () loop instead of a for () loop:
This works:
While this does not, and just gives me 100 instances of "fizzbuzz":
Also, I thought of placing the "var x = 0" after the divisibleBy variables - this just counts from 1 - 100.
What sort of "logic" (not in the programming sense, in the layman's terms sense) am I missing here?
If we're able to define variables out of scope, and then call on them within our loop, how come this doesn't work?