Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial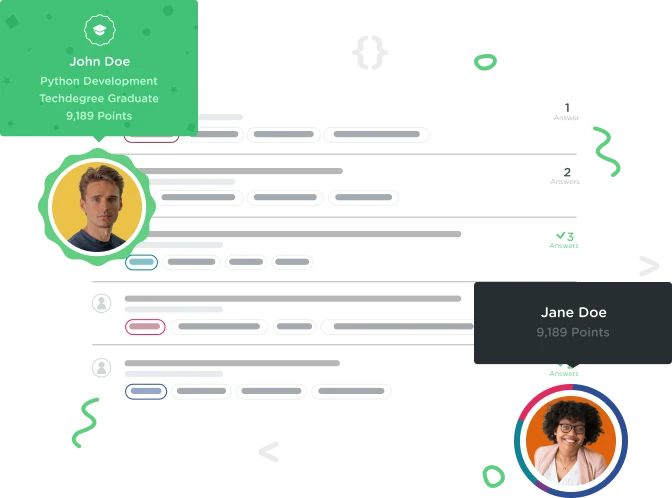

Amy Preci
6,247 PointsDefining variables outside of loops
Why do we always start with a variable defined outside of loops? For example "let counter = 0" why not within the loop? There was a quiz question that was similar, "let secret;" was declared outside of the loop. Thanks
1 Answer
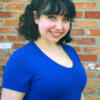
Bella Bradbury
Front End Web Development Techdegree Graduate 32,790 PointsHi Amy!
This has to do with Javascript Scope. Basically, a variable defined outside of a function is in the global scope, meaning it can be accessed anywhere in your code. This means that we can declare the variable outside of the function, but still access it anywhere in that file. Ex:
let message = "Hello there!";
function myMessage() {
message = "Goodbye";
}
myMessage();
console.log(message);
Our message is changed from hello to goodbye.
You can and should declare a variable in a function if it's not needed anywhere else. However, if it's declared inside of a function it will not be able to be accessed outside of that function. This also means that we could re-use variable names without anything getting confused. Ex:
function addNumbers() {
const high = 6;
const low = 4;
let total = high + low;
console.log(total);
// console would log 10
}
addNumbers();
function subtractNumbers() {
const high = 6;
const low = 4;
let total = high - low;
console.log(total);
// console would log 2
}
subtractNumbers();
And these two functions can exist in the same code without issue. Hope this helps!