Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial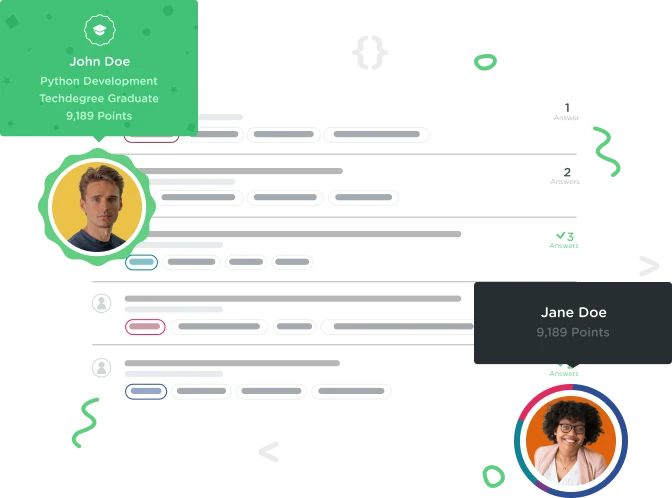

dlpuxdzztg
8,243 PointsDefinitions.
Could someone explain to me what primitive, literal, and string interning is in java? I'm pretty sure I have never covered this before...maybe I have...I dunno.
Thanks!
1 Answer
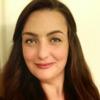
Jennifer Nordell
Treehouse TeacherWow you ask the tough ones, don't you? Well let's see if I can clarify some of it. A primitive data type is a data type that is predefined by the programming language. This is true of every programming language I've come across. You can find the java predefined data types here:
https://docs.oracle.com/javase/tutorial/java/nutsandbolts/datatypes.html
A string literal is simply a string in quotation marks. For example:
String name = "Diego";
The "Diego" part is the string literal. The type is String which happens to be a predefined type in Java thus making it a... you guessed it... primitive data type.
Now the string interning is interesting and it has to do with the heap or memory allocation. Let's say "Diego" appears 50 times through your code. As I understand it, what internalizing does is make it so that string has one memory location and every other reference of it points to that memory location instead of having 50 separate memory locations containg the same thing. But someone please tell me if I've misunderstood this!
If you read here and do a search on the page for "intern" the third hit you get will tell you a bit about the changes made in JDK 7 involving this. And they do mention the memory heap etc. http://www.oracle.com/technetwork/java/javase/jdk7-relnotes-418459.html
Hope this helps and that I didn't muddle it all up!
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsThank you very much!
markhunter4
5,564 Pointsmarkhunter4
5,564 PointsHi Jennifer,
Good response.
To clarify your response, Strings are not primitive type data! They are a class of data defined as an array of char (which is why char is lowercased and String is uppercase).
Intern, as described in the video is most useful for when you are dynamically instantiating a String, such as in
String mark = new String("mark")
or when you are taking user input (i.e. using a scanner). Interning adds the string into the string literal pool.
When declaring a String the normal way such as
String mark1 = "mark"
The Java automatically adds/references "mark" to the string pool at compile time (see 5:40 in video). Here's an example,
It is confusing, but it makes sense when you understand memory allocation.