Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial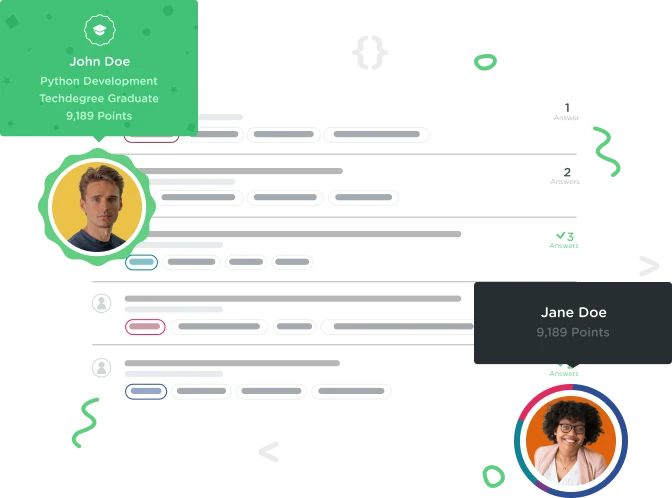

bogdan barbulescu
Courses Plus Student 232 PointsDelegate to SuperClass before initializing inherited properties
Am I right saying that when a property is inherited in a subclass, we have to delegate to a SuperClass designated initializer in order to avoid the case in which the designated initializer from the SuperClass would overwrite our property? Is this event happening due to the fact that classes are reference types and not value types.
class myVehicle{
var numberOfWheels = 0
var description: String {
return "\(numberOfWheels) wheel(s)"
}
}
let vehicle = myVehicle()
print(vehicle.description)
class myBicycle: myVehicle {
override init(){
super.init()
numberOfWheels = 2
}
}
let someBicycle = myBicycle()
print(someBicycle.numberOfWheels)
My understanding is that this code works in the following way. Let me know if I have understood it right.
- We are creating an instance of myBicycle() and the initializer from myBicycle subclass is called.
- Memory is allocated to create the instance.
- The overriden designated initializer init() ensures all stored properties introduced by the subclass ( there are none) are assigned/are initialized with an initial value.
- The overriden designated initializer init() now calls super.init()
- super.init() basically delegates to the SuperClass default initializer ( which is at all times a designated initializer)
- The default initializer from the SuperClass initializes its only stored property introduced by the class , var numberOfWheels = 0
- The memory of myBicycle() instance is considered fully initialized and each class is given the opportunity to further customize its properties, access self...etc.
- The computed
description
property returns a string - The customization moves down the chain to the subclass myBicycle
- numberOfWheels inherited property is initialized with a value of 2 by override init() initializer.