Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial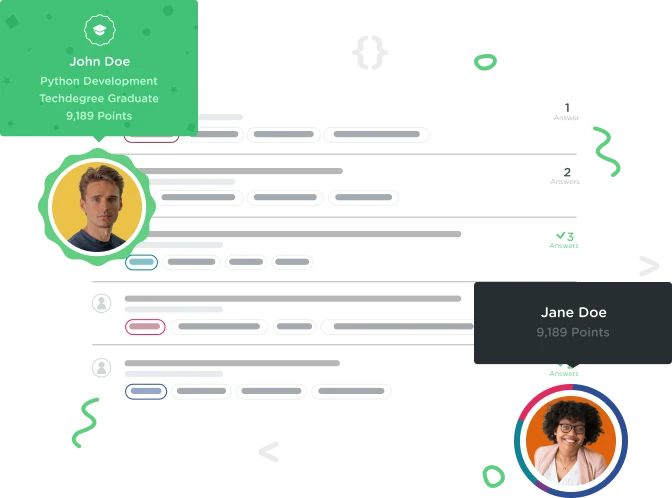

John Weland
42,478 PointsDelete Data in Angular
So I finished the MEAN course and enjoyed it. I noticed we never covered deleting things out of mongoDB with mongoose. I was going to tackle this but was curious to know if anyone else has done this. What was your approach?
2 Answers

Ken Howard
Treehouse Guest TeacherYou'll need to add a DELETE route on the apiRouter in express (in src/api/index.js)
apiRouter.delete('/todos/:id', function (req, res) {
var todoId = req.params.id; // This maps to the :id in the url
Todo.findByIdAndRemove(todoId, function (err, result) {
if (err) {
res.status(500).json({ message: err.message });
} else {
res.json({ message: 'Deleted Todo' });
}
});
});
Once the DELETE route is created you can use postman to send a DELETE HTTP request.. but you'd probably want to configure angular to do the delete when the user clicks the button. The button is already configured to fire the deleteTodo
method on dataService
. You just need to modify that a bit... (app/services/data.js)
this.deleteTodo = function(todo) {
if (!todo._id) {
return $q.resolve();
}
return $http.delete('/api/todos/' + todo._id).then(function () {
console.log("I deleted the " + todo.name + " todo!");
});
};
The way the TodoCtrl is currently set up is to remove the todo from the list before it calls the deleteTodo method on dataService. This is OK, but what if the server request fails? Change the deletedTodo method on the controller to look like this:
$scope.deleteTodo = function (todo, index) {
dataService.deleteTodo(todo).success(function () {
$scope.todos.splice(index, 1);
});
});
When the request is successful then we can safely remove the todo from the list.
I hope this helps. Let me know if you have any trouble implementing this.
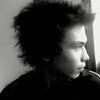
Deni Chan
Courses Plus Student 19,384 PointsI didnt implement this part
$scope.deleteTodo = function (todo, index) {
dataService.deleteTodo(todo).success(function () {
$scope.todos.splice(index, 1);
});
});
And it worked for me... But still when I mark todo as done its not presist
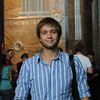
Dobrea Vladislav
14,141 PointsI have found why it does not persist, it is because in templates/todo.html you probably have
ng-init="todo.completed = false"
which overrides your persistent data from database;

John Weland
42,478 PointsDobrea Vladislav I noticed that too but I'm unsure how to fix it because removing that seems to no initialize things to false when you add new things.

John Weland
42,478 PointsDobrea Vladislav NVM I removed it with webpack running and it works... odd...

Daniel Cudney
4,515 Pointsadd this to main.js in controllers after you remove (ng-init="todo.completed = false") frin templates/todos.html
$scope.addTodo = function() {
console.log("Added");
var todo = {name: "Become the best version of you.", completed: false};
$scope.todos.unshift(todo);
};

Daniel Cudney
4,515 Pointsadd this to main.js in controllers after you remove (ng-init="todo.completed = false") frin templates/todos.html
$scope.addTodo = function() {
console.log("Added");
var todo = {name: "Become the best version of you.", completed: false};
$scope.todos.unshift(todo);
};
John Weland
42,478 PointsJohn Weland
42,478 PointsI implemented your method which was close to what I was attempting to do
I am recieving this output in the console when i delete an item (it DOES delete the items though)
Here are my files
Also when I complete and item and save it tells me it saves and the JSON reflects that Completed:true. However on page refresh that item is back to completed:false.
Ken Howard
Treehouse Guest TeacherKen Howard
Treehouse Guest TeacherJohn Weland ... replace the
.success
method with.then
method and it should work. The.success
method is only available on a raw promise. Sorry for the bad code.John Weland
42,478 PointsJohn Weland
42,478 PointsNo worries mate, changed it and it works like a charm. Now once I get the completed status to save, I'll be golden.
Plan is to extend this out to allow todo items to have categories. But one thing at a time I suppose. Thanks again.
Andrew Gursky
12,576 PointsAndrew Gursky
12,576 PointsI'm implementing this, but getting a 500 internal server error when I use it. Any insights?