Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial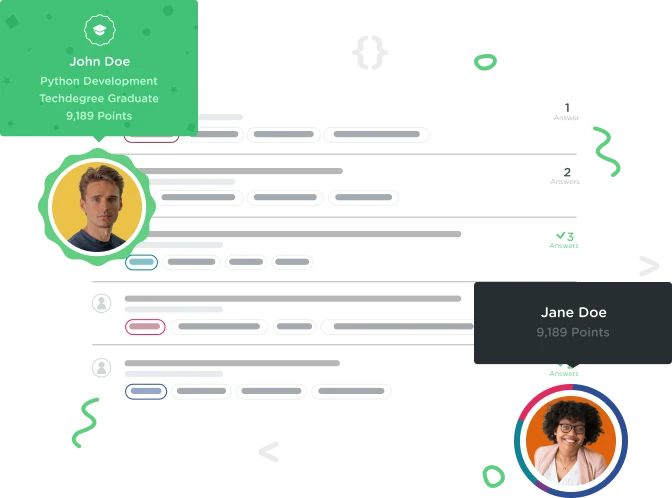

The Starlord
886 PointsdeselectRowAtIndexPath fails to deselect a row
even after executing [self.tableView deselectRowAtIndexPath:indexPath animated:NO]; in didDeselectRowAtIndexPath method app doesn't deselect the selected cell it remains highlighted.
xcode 6, iOS 8.3 SDK
//
// EditFriendsTableViewController.m
// Ribbit
//
#import "EditFriendsTableViewController.h"
#import <Parse/Parse.h>
@interface EditFriendsTableViewController ()
@end
@implementation EditFriendsTableViewController
- (void)viewDidLoad {
[super viewDidLoad];
PFQuery * query = [PFUser query];
[query orderByAscending:@"username"];
[query findObjectsInBackgroundWithBlock:^(NSArray * objects, NSError * error){
if (error) {
NSLog(@"the error %@, %@",error,error.userInfo);
}else{
self.allusers = objects;
[self.tableView reloadData];
self.currentUser = [PFUser currentUser];
}
}];
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
// Return the number of rows in the section.
return self.allusers.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"cell" forIndexPath:indexPath];
PFUser * user = [self.allusers objectAtIndex:indexPath.row];
cell.textLabel.text = user.username;
return cell;
}
-(void)tableView:(UITableView *)tableView didDeselectRowAtIndexPath:(NSIndexPath *)indexPath{
[self.tableView deselectRowAtIndexPath:indexPath animated:YES];
UITableViewCell * cell = [tableView cellForRowAtIndexPath:indexPath];
cell.accessoryType = UITableViewCellAccessoryCheckmark;
PFRelation * friendsRelation = [self.currentUser relationForKey:@"friendsRelation"];
PFUser * user = [self.allusers objectAtIndex:indexPath.row];
[friendsRelation addObject:user];
[self.currentUser saveInBackgroundWithBlock:^(BOOL succeeded, NSError * error){
if (error) {
NSLog(@"error %@, %@", error,error.userInfo);
}
}];
}
/*
// Override to support conditional editing of the table view.
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath {
// Return NO if you do not want the specified item to be editable.
return YES;
}
*/
/*
// Override to support editing the table view.
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath {
if (editingStyle == UITableViewCellEditingStyleDelete) {
// Delete the row from the data source
[tableView deleteRowsAtIndexPaths:@[indexPath] withRowAnimation:UITableViewRowAnimationFade];
} else if (editingStyle == UITableViewCellEditingStyleInsert) {
// Create a new instance of the appropriate class, insert it into the array, and add a new row to the table view
}
}
*/
/*
// Override to support rearranging the table view.
- (void)tableView:(UITableView *)tableView moveRowAtIndexPath:(NSIndexPath *)fromIndexPath toIndexPath:(NSIndexPath *)toIndexPath {
}
*/
/*
// Override to support conditional rearranging of the table view.
- (BOOL)tableView:(UITableView *)tableView canMoveRowAtIndexPath:(NSIndexPath *)indexPath {
// Return NO if you do not want the item to be re-orderable.
return YES;
}
*/
/*
#pragma mark - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
// Get the new view controller using [segue destinationViewController].
// Pass the selected object to the new view controller.
}
*/
@end
6 Answers
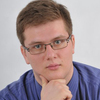
Cristian Malita
4,103 PointsYou are implementing the wrong method, didDeselectRowAtIndexPath instead of didSelectRowAtIndexPath. I made the same mistake, since the method names look very similar and didDeselectRowAtIndexPath comes first in the Xcode autocomplete.

The Starlord
886 PointsAppreciate your help, i tried it first with "NO" like it said in tutorial but dint help.
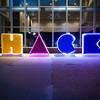
arshin
17,770 PointsPlease migrate the code under "didSelectRowAtIndexPath" to a Boolean method returning false, namely: "shouldHighlightRowAtIndexPath".
Feel free to search the documentation for declaration guidelines or use the auto complete feature in XCode.

The Starlord
886 Pointsyes that was my guess too, thank you for followingup appreciate it.
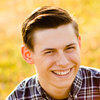
Caleb Kleveter
Treehouse Moderator 37,862 PointsIt is possible that you need different code for the new iOS SDK, as that tutorial is old in tech years.
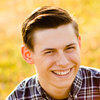
Caleb Kleveter
Treehouse Moderator 37,862 PointsI found this line in your code:
[self.tableView deselectRowAtIndexPath:indexPath animated:YES];
Change animated to NO.
Madhav Sharma
4,081 PointsMadhav Sharma
4,081 PointsWELL SPOTTED! thanks!