Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial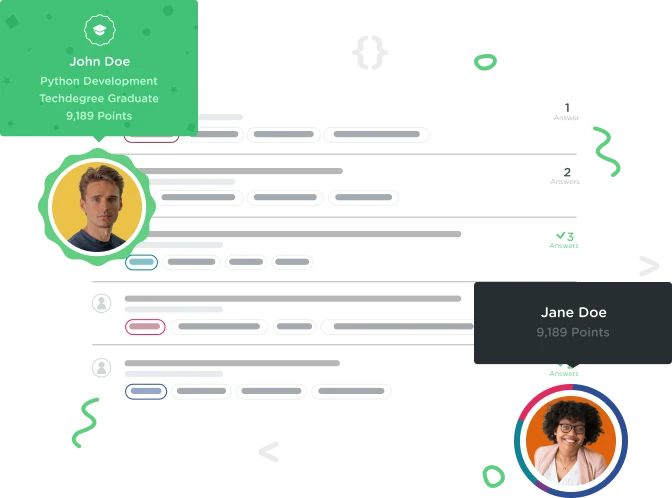
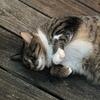
Steve Agusta
6,221 PointsDeserialize JSON to objects: having trouble with my own project
I'm trying to write a console application to send a GET request to an API and parse out the results, similar to what was shown in the course, but I'm stuck. The API I'm using is the USGS Earthquake Catalog: https://earthquake.usgs.gov/fdsnws/event/1/ It returns data on earthquakes in a given date range in JSON format. I've got the Webclient query and am getting the results I should be seeing. However, the response is a bit different to the example shown in the course, so I'm stuck on how to create the list and deserialize the objects.
What I have so far:
using System; using System.Collections.Generic; using System.IO; using System.Net; using Newtonsoft.Json;
namespace Earthquakes { class Program { static void Main(string[] args) { GetEarthquakes(); }
public static void GetEarthquakes()
{
var webClient = new WebClient();
byte[] earthquakeBytes = webClient.DownloadData("https://earthquake.usgs.gov/fdsnws/event/1/query?format=geojson&starttime=2020-08-01&endtime=2020-08-02");
var serializer = new JsonSerializer();
using (var stream = new MemoryStream(earthquakeBytes))
using (var reader = new StreamReader(stream))
using (var jsonReader = new JsonTextReader(reader))
{
Console.WriteLine(serializer.Deserialize<Properties>(jsonReader).Magnitude);
Console.WriteLine(serializer.Deserialize<Properties>(jsonReader).Place);
}
}
}
}
And my class file:
using System; using System.Collections.Generic; using System.Text; using Newtonsoft.Json;
namespace Earthquakes {
public class EarthquakeFeed
{
public string type { get; set; }
public Metadata metadata { get; set; }
public Feature[] features { get; set; }
public float[] bbox { get; set; }
}
public class Metadata
{
public long generated { get; set; }
public string url { get; set; }
public string title { get; set; }
public int status { get; set; }
public string api { get; set; }
public int count { get; set; }
}
public class Feature
{
public string type { get; set; }
public Properties properties { get; set; }
public Geometry geometry { get; set; }
public string id { get; set; }
}
public class Properties
{
[JsonProperty(PropertyName = "mag")]
public float Magnitude { get; set; }
[JsonProperty(PropertyName = "place")]
public string Place { get; set; }
public long time { get; set; }
public long updated { get; set; }
public object tz { get; set; }
public string url { get; set; }
public string detail { get; set; }
public int? felt { get; set; }
public float? cdi { get; set; }
public float? mmi { get; set; }
public string alert { get; set; }
public string status { get; set; }
public int tsunami { get; set; }
public int sig { get; set; }
public string net { get; set; }
public string code { get; set; }
public string ids { get; set; }
public string sources { get; set; }
public string types { get; set; }
public int? nst { get; set; }
public float? dmin { get; set; }
public float rms { get; set; }
public float? gap { get; set; }
public string magType { get; set; }
public string type { get; set; }
public string title { get; set; }
}
public class Geometry
{
public string type { get; set; }
public float[] coordinates { get; set; }
}
}