Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial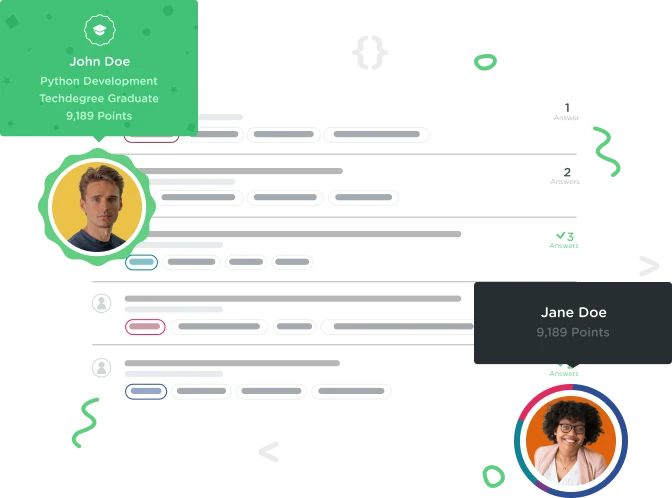

Victoria M
8,730 Pointsdesignated initializer task
The task is asking to figure out why the designated initializer isn't working and I tried re working the last part of the initializer but I still can't get it to work. Any ideas? Thank you!
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func scaleBy(points: Double){
width += points
height += points
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
init(width: Double, height: Double, cornerRadius: Double){
self.cornerRadius = cornerRadius
super.init(width: Double, height: Double)
}
}
1 Answer

Martin Wildfeuer
Courses Plus Student 11,071 PointsYou are basically on the right track. You have to call super.init
to set the initial values for width
and height
.
However, you have to pass the actual values when calling the initializer. You passing the type "Double" instead of the width/height value, which is an error that should be highlighted in XCode.
Therefore
super.init(width: Double, height: Double)
should be
super.init(width: width, height: height)
In context, you can see that you set the corner radius in the initializer of RoundButton
, and then pass on width and height to the designated initializer of the Button
superclass.
init(width: Double, height: Double, cornerRadius: Double){
self.cornerRadius = cornerRadius
super.init(width: width, height: height)
}
}
Hope that helps!
Victoria M
8,730 PointsVictoria M
8,730 PointsThat helps a lot, thank you!