Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial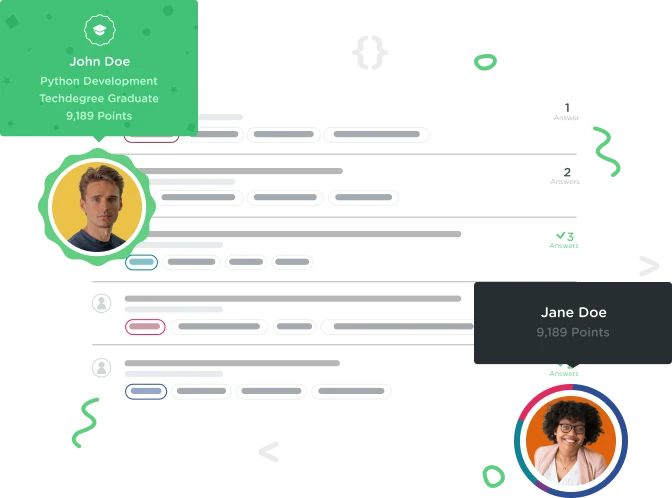

Scott Taylor
7,089 PointsDesigning and Starting the App - Challenge 2/3
I'm probably being very stupid but where am I going wrong with this code?
Question: The tabs are stored in an array named tabBarItems (line 9). Declare a UITabBarItem variable named tab and set it from tabBarItems using the selectedIndex property.
#import "MainViewController.h"
#import "UITabBarItem.h"
@implementation MainViewController
- (void)viewDidLoad {
[super viewDidLoad];
NSArray *tabBarItems = self.tabBarController.tabBar.items;
// Add your code below! The property for MainViewController's
// tab bar controller is named 'tabBarController'.
[self.tabBarController setSelectedIndex:2];
UITabBarItem *tab = [tabBarItems objectAtIndex:selectedIndex];
}
@end
8 Answers
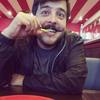
Dave Ko
11,915 PointsUITabBarItem *tab = tabBarItems [self.tabBarController.selectedIndex];

Stone Preston
42,016 Pointsyou are very close. Here is a hint: selectedIndex is a property of the tabBarController, so just using
[tabBarItems objectAtIndex:selectedIndex];
wont work. you have to chain dot notations together and access the tabBarController THEN the selectedIndex property of that tabBarController

Scott Taylor
7,089 PointsStone, thanks for helping me, I really appreciate it. I must be having a blonde moment, as I just can't get it. Any other advice or references you recommend I read? Thanks!

Stone Preston
42,016 Pointsok, you accessed the tabBarController in the first line you wrote:
[self.tabBarController setSelectedIndex:2];
now you have to access the selectedInexProperty of that same tabBarController using dot notation. Here is a hint: I can access that tabBar property of the tabBarController like so for example:
self.tabBarController.tabBar
you have to access the selectedIndex property of the tabBarController, not the tabBar property.

Scott Taylor
7,089 PointsThanks Stone, I got it (eventually!).
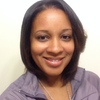
Sakina Burch
1,853 PointsKeep getting this error message There is a compiler error. Please click on preview to view your syntax errors! This is my Code UITabBarItem *tab = [self.selectedIndex.tabBarItems];
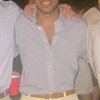
Nkem Modu
4,511 PointsI was having some trouble with this as well, but I finally got it. I'll try to break it down.
You are being asked to create a new UITabBarItem named "tab", and the value of "tab" will equal the value of an object in the "tabBarItems" array.
As we already know, we can access an object in an array if we know the index at which that object is stored:
array_name [index_number];
The array you have to access is "tabBarItems". The index number you want to access is stored within the variable "selectedIndex". However, you can't access "selectedIndex" directly; it is a property of "tabBarController" (and "tabBarController is a property of self).
You know the name of the array.
You know the dot notation that represents the index number.

wenwen liao
5,736 PointsI have a hard time too, but finally make it! here is my code:
UITabBarItem *tab = tabBarItems[self.tabBarController.selectedIndex];