Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial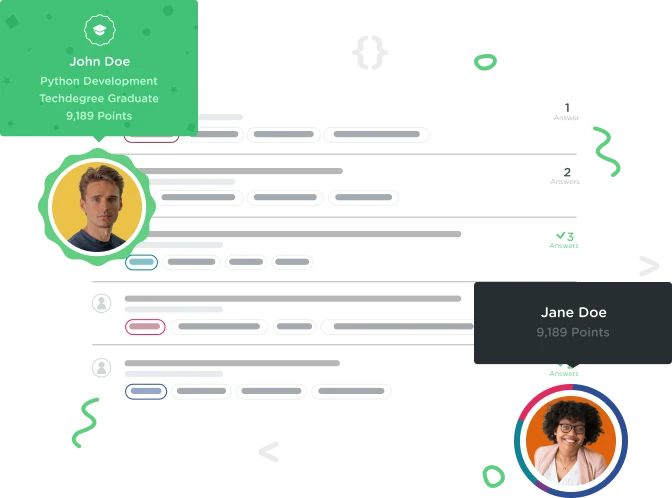
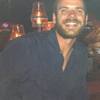
Aaron Brown
2,551 PointsDevoweling the vowelful using user input. What the heck is wrong with my code?
I am attempting to write my own version of the devowel.py program that Kenneth wrote. I did a lot of Googling and research on Stack Overflow to get where I currently am. The program runs without kicking errors, but unfortunately my while loop runs infinitely and it does not remove the vowels.
When I run it piece by piece in the interpreter my bool methods work. However, since my loop isn't ending, I'm assuming it's poor looping on my part. Does anyone see what may be the cause?
>>> input_word = input("Please enter any word, and we'll get rid of those pesky vowels: ")
Please enter any word, and we'll get rid of those pesky vowels: trhs
>>> vowels_bool = bool(vowels.intersection(input_word))
>>> vowels_bool
False
>>> input_word = input("Please enter any word, and we'll get rid of those pesky vowels: ")
Please enter any word, and we'll get rid of those pesky vowels: treehouse
>>> vowels_bool = bool(vowels.intersection(input_word))
>>> vowels_bool
True
In addition to the looping error. My remove vowels set does not work. I did some digging and it looks like it should work, but it's not.
I appreciate all constructive criticism!
Disclaimer: I know the code is ugly.
# I want the user to enter a word
input_word = input("Please enter any word, and we'll get rid of those pesky vowels: ")
# I want to tell the computer what a vowel is
vowels = set(['a', 'e', 'i', 'o', 'u', 'y'])
# I want to find out if my word has vowels
vowels_bool = bool(vowels.intersection(input_word))
# I need to convert user input to list form
input_word_list = list(input_word)
# I want to remove the vowels
vowels_removed = [v for i, v in enumerate(input_word_list) if i not in vowels]
# I want to print the word without the vowels and the first word capitalized
vowels_removed_string = ''.join(vowels_removed).capitalize()
while vowels_bool == True:
try:
vowels_removed = [v for i, v in enumerate(input_word_list) if i not in vowels]
except:
if vowels_bool == False:
print("Here's your vowelless word: {}".format(vowels_removed_string))
break
2 Answers

Dan Johnson
40,533 PointsYour vowels_bool variable never gets updated in the loop so it will always remain True
.
In your list comprehensions you're checking to see if the index/count is in vowels instead of a letter, as enumerate generates a list of tuples in the form (count, value)
.
Using list comprehensions you can do the following:
VOWELS = ['a', 'e', 'i', 'o', 'u']
response = input("Enter a word: ")
result = ''.join([letter for letter in response if letter not in VOWELS])
print(result)
Or a similar way that I find easier to read:
VOWELS = ['a', 'e', 'i', 'o', 'u']
response = input("Enter a word: ")
result = filter(lambda letter: letter not in VOWELS, response)
print(result)

Alan Dsouza
10,998 PointsSince you only need to check each letter in the word once, I recommend using 'for .. ' instead of a while loop. Here's how I would do it:
# I want the user to enter a word and instantly turn it into a list
input_word = list(raw_input('What is your name? '))
# I want to tell the computer what a vowel is
vowels = ['A', 'E', 'I', 'O', 'U', 'Y']
#function to loop over each letter in the word. If it is in the vowel list, remove it.
def remover(word, vowel_list):
for letter in word:
if letter.upper() in vowel_list:
word.remove(letter)
return ''.join(word).capitalize()
#run the function
devoweled = remover(input_word, vowels)
#print("Here's your vowelless word: {}".format())
print("Here's your vowelless word: {}".format(devoweled))
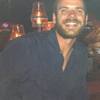
Aaron Brown
2,551 PointsHey, Alan! Thank you for your program. I like that you immediately turned the string into a list: of course!
Aaron Brown
2,551 PointsAaron Brown
2,551 PointsThanks, Dan, for such a quick, concise response. What a simple and elegant solution!