Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial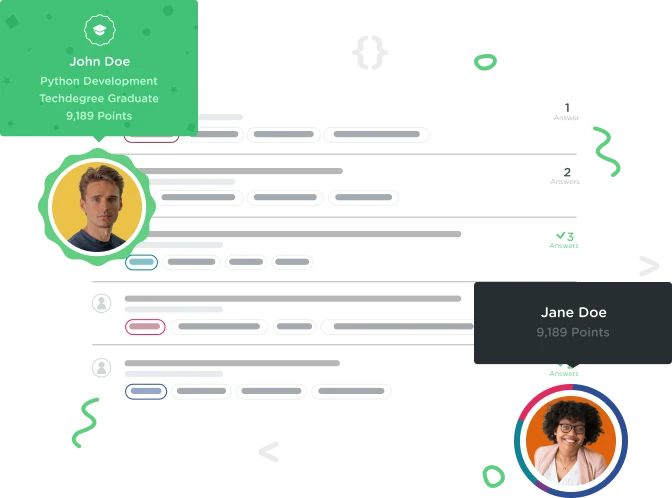
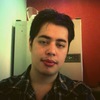
Jonathan Fernandez
8,325 PointsDiary App: pickedMood is incompatible... Compiler Error
I'm have a compiler error issue with my diary app regarding the pickedMood that was set.
I'm receiving a couple of warnings as well. Basically I can't figure out why I'm getting these warnings. I tried even changing the entity types. and remaking the subclass.
Yellow Warnings: Incompatible pointer to integer conversion assigning to 'int16_t' (aka 'short') from 'enum JWFDiaryEntryMood *'
Red Warnings: Statement requires expression of integer type ('enum JWFDiaryEntryMood *' invalid)
I would imagine they would want my mood to be an integer but doesn't an enum value return an integer? Or is it because it is an NS_ENUM and it's different from a regular enum?
Shown below is my code:
<p>
// Managed Object Subclass
//.h
#import <Foundation/Foundation.h>
#import <CoreData/CoreData.h>
// Declared Mood
NS_ENUM(int16_t, JWFDiaryEntryMood) {
JWFDiaryEntryMoodGood = 0,
JWFDiaryEntryMoodAverage = 1,
JWFDiaryEntryMoodBad = 2
};
@interface JWFDiaryEntry : NSManagedObject
@property (nonatomic) NSTimeInterval date;
@property (nonatomic, retain) NSString * body;
@property (nonatomic, retain) NSData * imageData;
@property (nonatomic) int16_t mood;
@property (nonatomic, retain) NSString * location;
@end
</p>
Shown below is my implementation on the entry view controller. It's got yellow marks all over and I have marked them with comments:
<p>
#import "JWFEntryViewController.h"
#import "JWFDiaryEntry.h"
#import "JWFCoreDataStack.h"
@interface JWFEntryViewController ()
@property (strong, nonatomic) IBOutlet UITextField *textField;
@property (nonatomic, assign) enum JWFDiaryEntryMood *pickedMood;
@property (weak, nonatomic) IBOutlet UIButton *badButton;
@property (weak, nonatomic) IBOutlet UIButton *averageButton;
@property (weak, nonatomic) IBOutlet UIButton *goodButton;
@property (strong, nonatomic) IBOutlet UIView *accessoryView;
@property (weak, nonatomic) IBOutlet UILabel *dateLabel;
@end
@implementation JWFEntryViewController
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date;
if (self.entry != nil) {
self.textField.text = self.entry.body;
self.pickedMood = self.entry.mood; //Warning Incompatible integer to pointer conversion assigning to 'enum JWFDiaryEntryMood*' from 'int16_t (aka 'short')
date = [NSDate dateWithTimeIntervalSince1970:self.entry.date];
} else {
self.pickedMood = JWFDiaryEntryMoodGood;
date = [NSDate date];
}
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"EEEE MMMM d, yyyy"];
self.dateLabel.text = [dateFormatter stringFromDate:date];
self.textField.inputAccessoryView = self.accessoryView;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void) insertDiaryEntry {
JWFCoreDataStack *coreDataStack = [JWFCoreDataStack defaultStack];
JWFDiaryEntry *entry = [NSEntityDescription insertNewObjectForEntityForName:@"JWFDiaryEntry" inManagedObjectContext:coreDataStack.managedObjectContext];
entry.body = self.textField.text;
entry.date = [[NSDate date] timeIntervalSince1970];
entry.mood = self.pickedMood; //Warning Incompatible integer to pointer conversion assigning to 'enum JWFDiaryEntryMood*' from 'int16_t (aka 'short')
[coreDataStack saveContext];
}
- (void)dismissSelf {
[self.presentingViewController dismissViewControllerAnimated:YES completion:nil];
}
- (void)updateDiaryEntry {
self.entry.body = self.textField.text;
self.entry.mood = self.pickedMood; //Warning Incompatible integer to pointer conversion assigning to 'enum JWFDiaryEntryMood*' from 'int16_t (aka 'short')
JWFCoreDataStack *coreDataStack = [JWFCoreDataStack defaultStack];
[coreDataStack saveContext];
}
- (void)setPickedMood:(enum JWFDiaryEntryMood *)pickedMood {
_pickedMood = pickedMood;
self.badButton.alpha = 0.5f;
self.averageButton.alpha = 0.5f;
self.goodButton.alpha = 0.5f;
// My code won't compile because switch won't accept pickedMood.. : (
switch (pickedMood) { //Statement requires expression of integer type ('enum JWFDiaryEntryMood *' invalid)
case JWFDiaryEntryMoodGood:
self.goodButton.alpha = 1.0f;
break;
case JWFDiaryEntryMoodAverage:
self.averageButton.alpha = 1.0f;
break;
case JWFDiaryEntryMoodBad:
self.badButton.alpha = 1.0f;
break;
}
}
#pragma mark - IBActions
- (IBAction)doneWasPressed:(id)sender {
if (self.entry != nil) {
[self updateDiaryEntry];
} else {
[self insertDiaryEntry];
}
[self dismissSelf];
}
- (IBAction)cancelWasPressed:(id)sender {
[self dismissSelf];
}
- (IBAction)badWasPressed:(id)sender {
self.pickedMood = JWFDiaryEntryMoodBad; //Warning Incompatible integer to pointer conversion assigning to 'enum JWFDiaryEntryMood*' from 'int16_t (aka 'short')
}
- (IBAction)averageWasPressed:(id)sender {
self.pickedMood = JWFDiaryEntryMoodAverage; //Warning Incompatible integer to pointer conversion assigning to 'enum JWFDiaryEntryMood*' from 'int16_t (aka 'short')
}
- (IBAction)goodWasPressed:(id)sender {
self.pickedMood = JWFDiaryEntryMoodGood; // For some strange reason this does not have a warning.. : /
}
@end
</p>
If anyone has any idea what's going on I would really appreciate your feedback!!
2 Answers
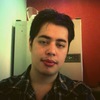
Jonathan Fernandez
8,325 PointsAfter going crazy for hours.. Googling stuff, even up to remaking the entities, and just staring at the code.. I had finally found an answer to my problem which made me want to face palm hard.. ><
Basically I just have to remove the * from my enum code. It's just that you tend to get so used to adding * to NS code that you forget about the basic things. XD
I have changed:
<p>
@property (nonatomic, assign) enum JWFDiaryEntryMood *pickedMood;
//...
// ......setPickedMood method
- (void)setPickedMood:(enum JWFDiaryEntryMood *)pickedMood {
</p>
to this..
<p>
@property (nonatomic, assign) enum JWFDiaryEntryMood pickedMood;
//...
// Removing * in setPickedMood method as well..
- (void)setPickedMood:(enum JWFDiaryEntryMood)pickedMood {
</p>
I'm leaving this answer here for reference in case anyone is as clumsy as me and does this by accident.
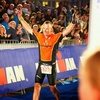
Steve Hunter
57,712 PointsTHANK YOU!!!
I had the same thing and it was driving me mad!!
Steve.
Alex Danson
8,737 PointsAlex Danson
8,737 PointsThanks for sharing your pain, I had the same issue and you've saved me a lot of time :)
Eduardo Mejía
36,816 PointsEduardo Mejía
36,816 PointsThank you so much Jonathan!!!