Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial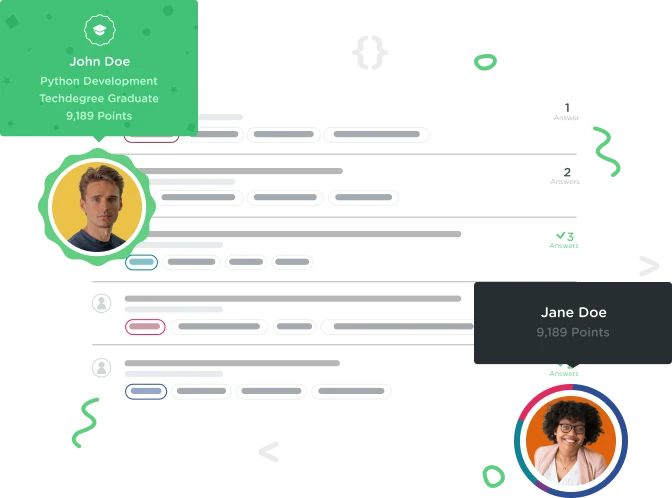

Dawid Chojnacki
5,977 PointsDiaryApp: Variable has incomplete type 'enum DiaryEntryMood' Compiler Error
I had the same issue like Jonathan Fernandez with incompatible type compiler error. I've deleted '*' from my enum code, but I'm still getting an error, expect this time it's "Variable has incomplete type 'enum DiaryEntryMood'". Maybe anyone know the answer? I spend one week on looking for the solution but nothing helps :/ I guess the solution is pretty simple but still it seems like I'm getting tired from this code because I can't figure out anything more. I put my code below:
Enum declaration:
#import "DiaryEntry.h"
NS_ASSUME_NONNULL_BEGIN
enum {
good = 0,
average = 1,
bad = 2
};
typedef int16_t DiaryEntryMood;
@interface DiaryEntry (CoreDataProperties)
@property (nonatomic) NSTimeInterval date;
@property (nullable, nonatomic, retain) NSString *body;
@property (nullable, nonatomic, retain) NSData *imageData;
@property (nonatomic) int16_t mood;
@property (nullable, nonatomic, retain) NSString *location;
@property (nonatomic, readonly) NSString *sectionName;
@end
NS_ASSUME_NONNULL_END
Header file of entry view controller:
#import <UIKit/UIKit.h>
@class DiaryEntry;
@interface AddPostViewController : UIViewController
@property (weak, nonatomic) IBOutlet UITextField *textField;
@property (strong, nonatomic) DiaryEntry *entry;
@property (nonatomic, assign) enum DiaryEntryMood pickedMood;
@property (weak, nonatomic) IBOutlet UIButton *badButton;
@property (weak, nonatomic) IBOutlet UIButton *averageButton;
@property (weak, nonatomic) IBOutlet UIButton *goodButton;
@property (strong, nonatomic) IBOutlet UIView *accessoryView;
@end
Implementation file of entry view controller:
#import "AddPostViewController.h"
#import "CoreDataStack.h"
#import "DiaryEntry+CoreDataProperties.h"
@interface AddPostViewController ()
@end
@implementation AddPostViewController //Cannot synthesize property 'pickedMood' with incomplete type 'enum DiaryEntryMood'
- (void)viewDidLoad {
[super viewDidLoad];
if (self.entry != nil) {
self.textField.text = self.entry.body;
_pickedMood = self.entry.mood;
} else{
_pickedMood = good;
}
self.textField.inputAccessoryView = self.accessoryView;
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void) insertDiaryEntry{
CoreDataStack *coreDataStack = [CoreDataStack defaultStack];
DiaryEntry *diaryEntry = [NSEntityDescription insertNewObjectForEntityForName:@"DiaryEntry" inManagedObjectContext:coreDataStack.managedObjectContext];
diaryEntry.body = self.textField.text;
diaryEntry.mood = _pickedMood;
diaryEntry.date = [[NSDate date] timeIntervalSince1970];
[coreDataStack saveContext];
}
- (void) updateDiary{
self.entry.body = self.textField.text;
self.entry.mood = _pickedMood;
CoreDataStack *coreDataStack = [CoreDataStack defaultStack];
[coreDataStack saveContext];
}
-(void)setPickedMood:(enum DiaryEntryMood)pickedMood { //Variable has incomplete type 'enum DiaryEntryMood'
_pickedMood = pickedMood;
self.badButton.alpha = 0.5;
self.averageButton.alpha = 0.5;
self.goodButton.alpha = 0.5;
switch (pickedMood) {
case bad:
self.badButton.alpha = 1.0;
break;
case average:
self.averageButton.alpha = 1.0;
break;
case good:
self.goodButton.alpha = 1.0;
break;
default:
break;
}
}
- (void) dismissSelf{
[self.presentingViewController dismissViewControllerAnimated:true completion:nil];
}
- (IBAction)doneButtonPressed:(id)sender {
if (self.entry != nil) {
[self updateDiary];
} else{
[self insertDiaryEntry];
}
[self dismissSelf];
}
- (IBAction)cancelButtonPressed:(id)sender {
[self dismissSelf];
}
- (IBAction)badWasPressed:(id)sender {
_pickedMood = bad;
}
- (IBAction)averageWasPressed:(id)sender {
_pickedMood = average;
}
- (IBAction)goodWasPressed:(id)sender {
_pickedMood = good;
}
@end
Pleeeeeeeeease does anybody know the solution? Thanks for answers! :D