Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial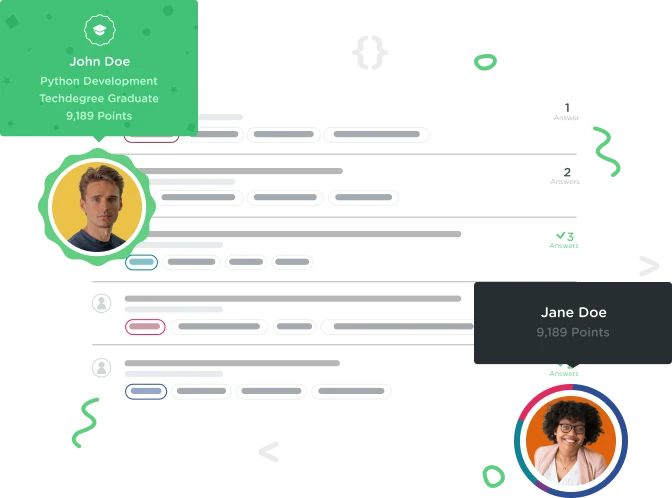
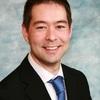
Mark Chesney
11,747 Pointsdict packing & unpacking (ref: Python Collections, favorite_food challenge)
Hi, I'm close but I haven't been able to resolve this error, nor do I have enough understanding of kwargs to know what the error may be. Here's the closest failing test case hint that I've received. Also, there is something about the challenge instructions that I'm certainly either misunderstanding or getting confused about. Thank you!
Here's the ERROR: Expected favorite_food({'name': 'Michelangelo', 'food': 'PIZZA'}) to return "Hi, I'm Michelangelo and I love to eat PIZZA!", but results instead were: "Hi, I'm {'name': 'Michelangelo', 'food': 'PIZZA'} and I love to eat None!"
def favorite_food(name=None, food=None):
return "Hi, I'm {} and I love to eat {}!".format(name, food)
5 Answers
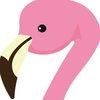
Dave StSomeWhere
19,870 PointsFirst of all kwargs is just a common term for keyword arguments and is just a variable name. It can be any valid variable name, even dict.
By placing ** in front of the dictionary name in a function it breaks out the name/value pairs (hence unpacking).
So, all the challenge only needs 1 small change which is to unpack the passed parameter in the .format() function (almost too easy)
Here's a SO explanation that might be useful - https://stackoverflow.com/questions/1769403/understanding-kwargs-in-python
Hope that helps.
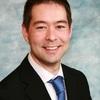
Mark Chesney
11,747 PointsI finally passed this challenge but it felt like through lots of random guesses. Surprised to find out that **kwargs was not involved in the solution, even though it's used for unpacking
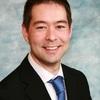
Mark Chesney
11,747 PointsThanks Dave. I resolved this, and found I had an incomplete understanding of packing and unpacking -- my incorrect understanding was that packing and unpacking always involves asterisks (** for dict and * for tuples), but I now know the assignment was asking for unpacking to be done without **. The difficult thing to grasp for me was that the use of "dict[name]" and "dict[food]" is considered unpacking -- I just thought of it as retrieving the value of a dict by passing the dict key through favorite_food(dict). So if that's incorrect, I welcome anyone's input. Alright, again, thank you!
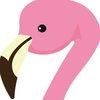
Dave StSomeWhere
19,870 PointsEither you're missing something or the challenge link is incorrect. When I look at the challenge it show the following: Original Challenge
def favorite_food(dict):
return "Hi, I'm {name} and I love to eat {food}!".format()
It that is the correct challenge then the solution is simply to use the ** (which is unpacking) on the passed parameter in the format function to break out and insert the name and food values. (why I said almost too simple) Solution
def favorite_food(dict):
return "Hi, I'm {name} and I love to eat {food}!".format(**dict)
I'm unaware of considering dict['name' ] as unpacking - I always thought of it as referencing - how you get the value from the keyword.
Does that make any sense?
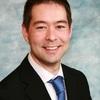
Mark Chesney
11,747 PointsWell, thank you sir. The link is correct, same challenge. I'm glad you replied because I learned a few things. Here is what I'm gathering from this conversation:
1) I can pass this challenge, by referencing rather than by unpacking. This means I can complete it without actually applying the concept from the lesson, because this is how I passed it: def favorite_food(dict): return "Hi, I'm {} and I love to eat {}!".format(dict['name'],dict['food'])
2) I see packing done in the parameters of the first "def" line, but I didn't know unpacking can be done after in line 2, as in the solution you've shown me. because my understanding is that unpacking is done in the function call, and packing is done in the function definition. Please correct me if I'm wrong about that.
I appreciated your hint that the solution was almost too simple. I don't know what to tell you, however. I suppose if I had known what you knew, then I would find it almost too simple as well :-)
Again thanks, now I know how I would have solved it for future reference.:-)
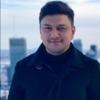
ARCHIT RANA
Full Stack JavaScript Techdegree Graduate 27,853 PointsI guess there is something wrong with the link.
This is the code that I ran:
my_dict = {"name" : "Michelangelo", "food" : "PIZZA"}
def favorite_food(name = "None", food = "None"): return "Hi, I'm {} and I love to eat {}!".format(name, food)
favorite_food(**my_dict)
- It ran in the IDE but showed error in the challenge.
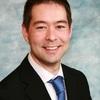
Mark Chesney
11,747 PointsHi Archit, seems like you're experiencing the same as I had.
You can "can pass this challenge, by referencing rather than by unpacking" (recycling my own words). I ran your code in my Python console and, like you, there was no error.
But if you can pass the dictionary as the one and only parameter in the func definition, then you can demonstrate dictionary unpacking. Best of luck!