Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial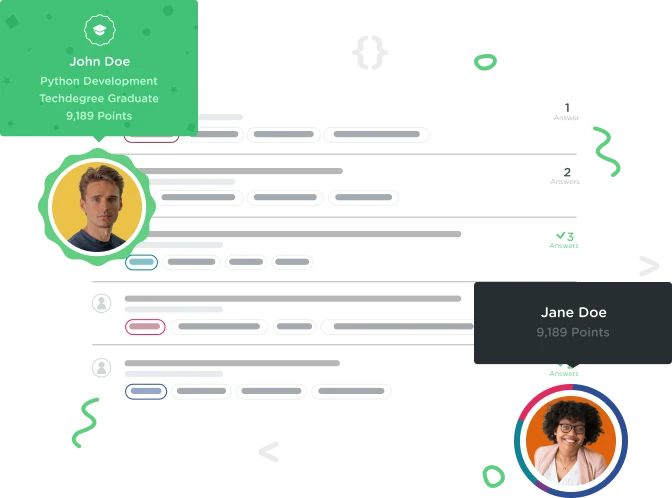

Idris Abdulwahab
Courses Plus Student 2,961 PointsDictionaries
Code challenge problem:
I know my code is wrong. My problem is how to count the number values in the dictionaries, including the values that are in a list.
Help with some hints and may be I could figure it out.
Thank you.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(dict):
new = dict
number = len(new.keys())
return (number)
def num_courses(dict):
plan = dict
for key, value in plan.items():
if len(value) > 1:
noom = len(value)
good = noom + len(plan.values())
else:
good = len(plan.values())
return (good)
6 Answers

Andrey Misikhin
16,529 PointsYou need to check type of value.
def num_courses(dictionary):
count = 0
for teacher, courses in dictionary.items():
if type(courses) is list:
count += len(courses)
else:
count += 1
return count

Andrey Misikhin
16,529 PointsI completed this task with using of tuples. Give you a hint:)
def num_courses(dict):
for item in dict.items():
#now in item you have a tuple like ('Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'])
#you may get name using index 0 and list of courses using index 1
#item[0] - name, len(item[1]) - number of courses for the teacher

Idris Abdulwahab
Courses Plus Student 2,961 PointsThank you for the hints but it's still not working as I want it.
For example:
def num_courses(dict):
for item in dict.items():
dd = len(item[1])
dd += dd
print (dd)
num_courses({'mike' : 'maths' , 'scot' : 'english' , 'glen' : ['social' , 'health'] , 'frank' : ['science' , 'trade']})
Two problems I encounter are:
len(item[1]) gives the correct number of courses for each teacher if [item] is a list. But if [item] is a string, it gives the number of characters in the string with is not what i want. So using len(item[1]) I get 2 courses each for glen and frank which is correct but I get 4 courses each for mike and scot which is WRONG. That is because len(item[1]) for the string item returns the number of characters in the string and does not count the whole string as one item.
Then how do i add up the total number of item[1] for all the items in dict.items(). I thought that dd+=dd should do it but it's not. So calling function as I did above, I should have a total of 6 courses for all the teachers.
Please help to clear this out.
Thank you.

Idris Abdulwahab
Courses Plus Student 2,961 PointsThanks for the last time. For the past three hours i've tried solving another step but cannot get through. This time around it's to know which teacher has the highest number of courses. Some of the courses are listed as before.
```def most_courses(dict): course_count = 0 for teacher, courses in dict.items(): if type(courses) is list: course_count = len(courses) else: course_count += 1 max_count = max(course_count) for teacher in dict.items(): print (teacher, max_count)
most_courses({'Dan' : 'econs' , 'Mike' : 'Phys' , 'Scot' : ['bio', 'stem', 'maths'] , 'Malo' : 'chem' , 'Bigi' : ['trade', 'selling']})
I got this error when i called the function:
Traceback (most recent call last):
File "dict_2.py", line 12, in <module>
most_courses({'Dan' : 'econs' , 'Mike' : 'Phys' , 'Scot' : ['bio', 'stem', 'maths'] , 'Malo' : 'chem' , 'Bigi' : ['trade', 'selling']})
File "dict_2.py", line 8, in most_courses
max_count = max(course_count)
TypeError: 'int' object is not iterable
Give me some hints and let me try again.
Thank you.

Bob Pickles
1,363 PointsThis is what worked for me:
def num_courses(teachers):
count=0
for course in teachers.values():
count += len(course)
return count
You know the data is in a list so you don't need to check for that (and even if it wasn't, the above mentioned code would just error out because there's no error handling). With that knowledge you can get only the values with dict.values(). This gives you the list of courses stored into the course variable for each loop. You can then count with len() and store that value in your increment counter (count).

Bob Pickles
1,363 PointsI am having a bit of an issue:
The challenge says to return a single list with all the available courses. I have this code which looks to return the correct answer in workspaces but it's failing in the challenge:
def courses(teachers):
new_list=[]
for course in teachers.values():
for c in course:
new_list.append(c)
return new_list
returns
['jQuery Basics', 'Node.js Basics', 'Python Basics', 'Python Collections']
Any thoughts on what I'm doing wrong?

Bob Pickles
1,363 PointsSo I found someone else's courses function and tried it. However, I don't understand why it works but mine doesn't. The output looks the same in workspaces.
My code
def courses(teachers):
new_list=[]
for course in teachers.values():
for c in course:
new_list.append(c)
return new_list
Their code
def courses(teach_dict):
class_list = []
for teacher in teach_dict:
for course in teach_dict[teacher]:
class_list.append(course)
return class_list
Output
['Python Basics', 'Python Collections', 'jQuery Basics', 'Node.js Basics'] <--theirs
['Python Basics', 'Python Collections', 'jQuery Basics', 'Node.js Basics'] <--mine

Bob Pickles
1,363 PointsOne more small bit of curiousity to add to this. I ran both functions in the same script then at the end did this
print(courses(teachers) == courses2(teachers)) thinking maybe there was something about the output that was different but not visual, nope, return was True
Idris Abdulwahab
Courses Plus Student 2,961 PointsIdris Abdulwahab
Courses Plus Student 2,961 PointsThank you so much Andrey. You got me out of the woods! My problem was the type. I appreciate your help on this.