Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial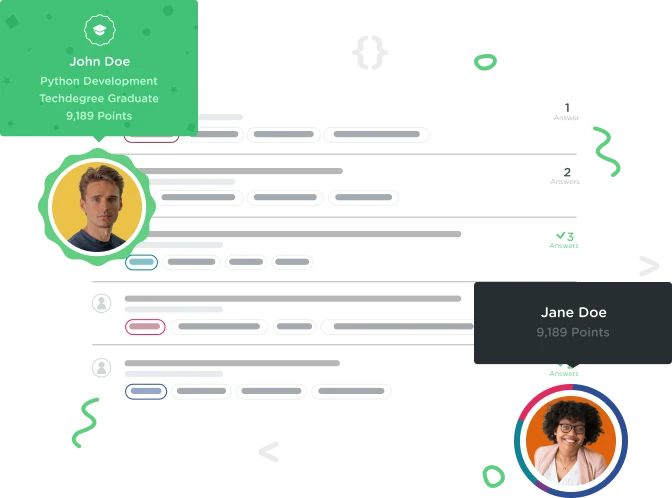
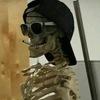
Ian Cole
454 PointsDictionaries and for loops hurt my soul
Honestly im completely lost for what im doing wrong at this point. Dictionaries confuse the living hell out of me and working them with for loops makes it even worse. I don't even know how half of this works, im suprised it works at all, especially the stuff in normal brackets. If anyone has any advice on this it would be much appreaciated
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(teacher_dict):
return len(teacher_dict)
def num_courses(teacher_dict):
course_total = 0
for teacher in teacher_dict:
for course in teacher_dict[teacher]:
course_total += 1
return course_total
def courses(teacher_dict):
course_list = []
for teacher in teacher_dict:
for course in teacher_dict[teacher]:
course_list.append(course)
return course_list
def most_courses(teacher_dict):
max_value = 0
for key in teacher_dict:
for course in teacher_dict[key]:
if len(course) > max_value:
max_value = len(course)
name = key
return name
1 Answer
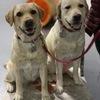
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHI Ian,
First, I'd like to reassure you that you're not alone. Many of us have found dictionaries to be one of the hardest data structures to get comfortable with. It really does get easier with practice though. In the meantime, don't be afraid to keep a little 'cheat sheet' of how to do common tasks with dictionaries. When you're learning a new language, there's too much to keep it all in your head at once.
As for your specific code here, you've almost got it. You just made it a little harder for yourself by getting the longest named course instead of the longest list of courses.
You don't need the inner loop, you just need to get the length of the value in teacher_dict at position key
(you've already successfully accessed that value to build your inner loop) and then update your max_value with that length.
For the future, there is another convenience syntax for dealing with both the key and the value when iterating through a dictionary. Instead of for key in dictionary
you can use for key, value in dictionary.items()
(maybe another thing for the cheat sheet) and then inside your loop you have direct access to the value as the value
variable instead of needing to get it from looking it up with the key each time dictionary[key]
.
Hope that helps,
Alex
Ian Cole
454 PointsIan Cole
454 PointsYou are a god. Thank you! This actually helped a ton!