Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial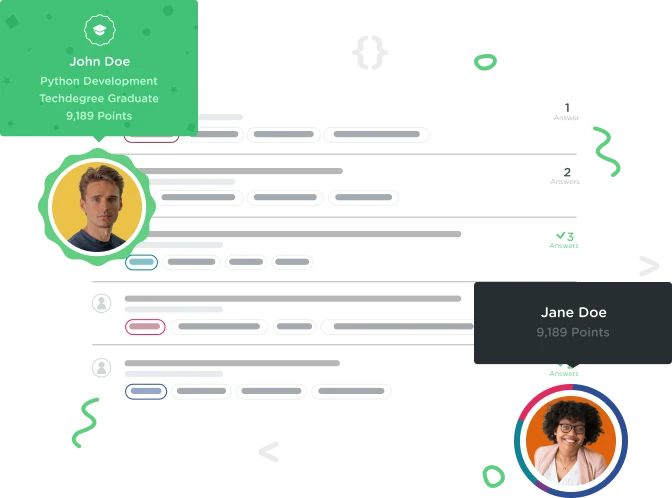
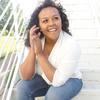
Enzie Riddle
Front End Web Development Techdegree Graduate 19,278 PointsDictionaries, Packing, and Unpacking
Here's the question:
Let's test unpacking dictionaries in keyword arguments. You've used the string .format() method before to fill in blank placeholders. If you give the placeholder a name, though, like in template below, you fill it in through keyword arguments to .format(), like this: template.format(name="Kenneth", food="tacos") Write a function named string_factory that accepts a list of dictionaries as an argument. Return a new list of strings made by using ** for each dictionary in the list and the template string provided.
What am I doing wrong? I've been following along with the last video, but I am honestly quite confused on packing and unpacking and the what's and why's of when we use them. Thanks!
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(name=None, food=None, second_name=None, second_food=None, **kwargs):
print(kwargs)
def unpacker(name=None, food=None, second_name=None, second_food=None):
if name and food and second_name and second_food:
print("Hi, I'm {} and I love to eat {}!".format(name, food) + " Hi, I'm {} and I love to eat {}!".format(second_name, second_food))
else:
print("Name and favorite food please!")
return unpacker(**{name: "Michelangelo", food: "PIZZA", second_name: "Garfield", second_food: "Lasagna"})
string_factory(name="Michelangelo", food="PIZZA", second_name="Garfield", second_food="Lasagna")
2 Answers
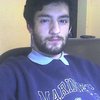
Dario Bahena
10,697 Points# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
#disclaimer - not sure if this is what is expected but it is the solution I came up with that works.
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(val):
tempelateList = [] #create an empty array
for i in val: # loop through the list provided like the one in the example of values
tempelateList.append(template.format(**i)) # **i is the keyword argument {"name": "Michelangelo", "food": "PIZZA"} on the first run
return tempelateList # returns an array with the correct strings
#string_factory(values)

Kamontat swasdikulavath
2,447 PointsI don't understand why does the 'i' work? Please explain

Afloarei Andrei
5,163 PointsI need some help. I've written this peace of coade
values = [{"name": "Andrei", "food": "pizza"}, {"name": "Ioana", "food": "hamburger"}] template = "Hi, I'm {} and I love to eat {}!"
def string_factory(name = None, food = None): if name and food: print(template.format(name, food)) else: print("Something went wrong!")
string_factory(values[0]) string_factory(values[1])
and if I try to add the result to a list I get this TypeError: string_factory() argument after ** must be a mapping, not list I don't know how to solve this one.
Juan Suarez
1,406 PointsJuan Suarez
1,406 PointsI did no look closely to the code but it seems that you are printing instead of returning, the code checker does not like that.