Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial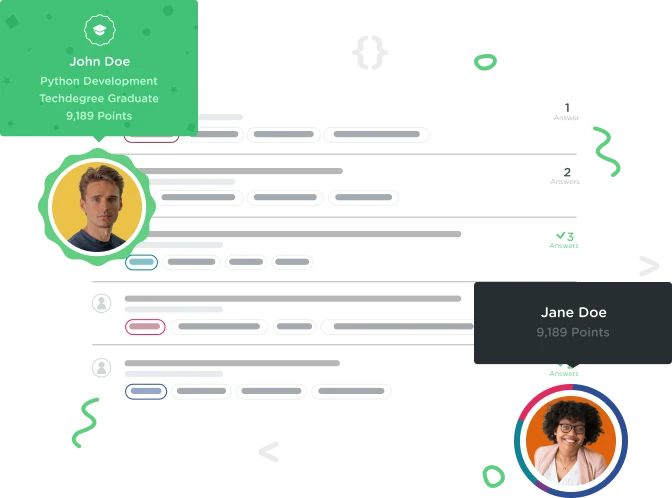

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 Pointsdictionary
can anyone help me to identify the error in following code:
def word_count(inp): str = inp.lower() inp_list = str.split() my_dict = dict() count = int(0) for i in inp_list: my_dict[inp_list[count]] = str.count(inp_list[count]) count = count+1 return my_dict
The above function should return the count of each words in the string as value in dictionary. In my local machine it produces expected result. But here it says there is some mismatch.?.
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(inp):
str = inp.lower()
inp_list = str.split()
my_dict = dict()
count = int(0)
for i in inp_list:
my_dict[inp_list[count]] = str.count(inp_list[count])
count = count+1
return my_dict
2 Answers

Konrad Kowalke
1,273 PointsHi there,
the solution is actually more simple than what you have in mind. All you need to do is iterate over the inp_list and save the count for each element in the dictionary:
for i in inp_list:
my_dict[i] = inp_list.count(i)
I also would avoid using "str" as a variable name, since it is a builtin function in python and might hurt readability of the code. Therefore it is seen as bad practice by some developers.
I hope I could help with your problem ;)

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsThank You very much. I don't that even in list , the method count() works. And again thank you for clarity on the variable "str". Everything worked well.