Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial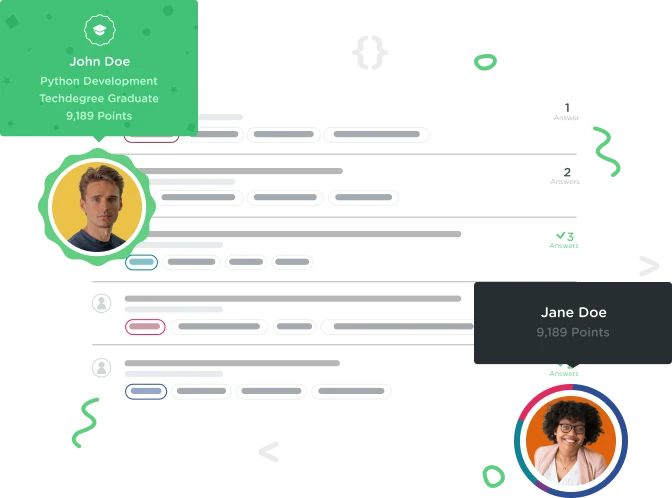
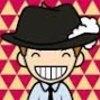
Aaron Selonke
10,323 PointsDictionary Challange
CHALLANGE DIRECTIONS Code the AddWord method such that, WordCount will contain the number of times the AddWord method has been called with a given word. For example, if AddWord is called twice with the word "rock" then WordCount["rock"] will be 2.
I thought that this was easy until I saw that the dictionary takes the string as the key and the integer as the value. And the code that I used below to increment the integer with every addition to the dictionary would not cut it.
I'm trying to understand how we can use the word rock twice as the key - as the challenge directions want, and not get a duplicate key error.
Thanks in advance for any replies
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
WordCount.Add(word, (WordCount.Count + 1));
}
}
}
2 Answers
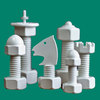
Steven Parker
231,545 Points
Clearly, you can't "Add" a specific key more than once.
You will want to see if the key already exists in the dictionary, and add it if it doesn't, but just increment the count if it does.

Toni Dzalto
21,667 PointsThe count property is not what you are looking for in this case, it "Gets the number of key/value pairs contained in the Dictionary<TKey,βTValue>."
First you have to check weather the key exists, and only use the Add function if it does not. However if it does exist you will want to increment WordCount[word] by 1.
public void AddWord(string word)
{
if(!WordCount.ContainsValue(word))
{
WordCount.Add(word, 1);
}else{
WordCount[word] = WordCount[word] + 1;
}
}

Sean Gibson
38,363 PointsDid you perhaps mean to say "WordCount.ContainsKey(word)"?