Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial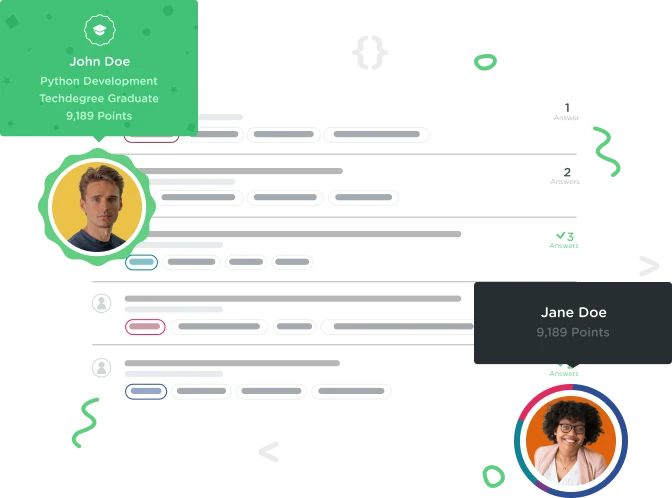

Ullas Savkoor
6,028 Pointsdictionary challenge word count-unable to find solution that passes the challenge
Challenge Task 1 of 1
Alright, this one might be a bit challenging but you've been doing great so far, so I'm sure you can manage it.
I need you to make a function named word_count. It should accept a single argument which will be a string. The function needs to return a dictionary. The keys in the dictionary will be each of the words in the string, lowercased. The values will be how many times that particular word appears in the string. PLEASE LET ME KNOW WHERE IS THE BUG IN CODE
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
string = string.lower()
word_dict = {}
word_list = string.split(" ")
for sel_word in word_list:
count = 0
for word in word_list:
if sel_word == word:
count +=1
word_dict[sel_word] = count
return word_dict
14 Answers
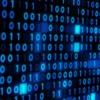
Alexander Davison
65,469 PointsYou need to change this line of code:
word_list = string.split(" ")
To this:
word_list = string.split()
The difference is that .split(" ")
splits only on spaces and .split()
splits on all whitespace. Whitespace includes spaces, tabs, newlines, vertical spaces, etc.
The challenge expects you to split on all whitespace.
Everything else in your code looks great and is very clean and easy-to-read. Congrats!
I hope this helps
Happy coding!
~Alex
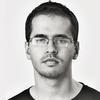
Jan Giemza
32,959 PointsI did it this way, with two simple for loops. Hopefully, this is easy to read and understand.
def word_count(string):
words = string.lower().split()
dictionary = {}
for word in words:
dictionary[word] = 0
for word in words:
dictionary[word] += 1
print(dictionary)
return dictionary
word_count("I do not like it Sam I Am")
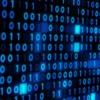
Alexander Davison
65,469 PointsI like your way :)
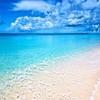
eodell
26,386 PointsI like yours than the others. Thanks a lot!!! Although the last part of
print(dictionary) return dictionary
It could be either one, right?
Thanks again :)

Sohail Mirza
Python Web Development Techdegree Student 5,158 PointsHi, could someone explain the above code by Jan. I want to know what know the following 1, What does each of the for in loop do in other others word what is it outputting 2, i don't understand the this [word] means

Mark Tripney
6,009 PointsThe count()
method makes this relatively straightforward. I've included some comments which might make things clearer...
def word_count(a_string):
# Convert 'a_string' into lower case and break it up into a list
words = a_string.lower().split()
# Declare an empty dictionary, ready to be populated
dictionary = {}
# For each item in the list, use the 'count()' method to tally how
# often it appears. Then, populate the dictionary with a key (the word)
# and its corresponding value (the count).
for word in words:
dictionary[word] = words.count(word)
return dictionary
print(word_count("I do not like it Sam I Am"))

Sai C
2,577 PointsI think yours is the best! super clear by using a single line to assign value (words.count) to keys(word)

Steven Pichardo
10,062 Pointsfound this to work also
import collections
def word_count(astring):
newstring = astring.lower().split()
return collections.Counter(newstring)
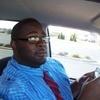
Christopher Bailey
14,880 PointsSame what I did,
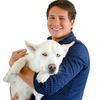
Cristian Romero
11,911 PointsLove this answer.. I think it's important to start thinking imports to make the work easier..

Ankit Pansari
6,208 Pointsdef word_count(string):
string = string.lower()
new_dict = {}
for i in string.split():
new_dict[i] = string.split().count(i)
return new_dict
I tried using the count method for list.
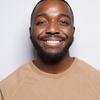
Jonathan Peters
8,672 PointsI love this solution, thanks for this!

Morningstar Ndlovu
3,732 Pointsthank you Pansari

Vlad Bitca
Courses Plus Student 2,702 PointsYou can try:
from collections import Counter
def word_count(sentence):
return Counter(map(str, sentence.lower().split()))

Denis Watida Machaka
7,311 Pointsthis one work smooth as well thanx

Sabin Boboc
2,587 Pointsdef word_count(proposition):
words = proposition.lower().split()
word_freq = {}
for w in words:
try:
word_freq[i] += 1
except KeyError as ke:
word_freq[i] = 1
return word_freq

Ullas Savkoor
6,028 PointsAlexander Davison :Request your help in finding the bug in my solution for word_count

Artsiom Nestsiarenka
2,438 Pointsthis code works. what kind of bug?

Ullas Savkoor
6,028 PointsAs Alex mentioned .split(" ") splits only on spaces and .split() splits on all whitespace, the code challenge is expecting for whitespaces and my code was only splitting on spaces.
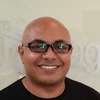
Michael Nessim
7,635 Pointsdef word_count(string): dic = {} array = string.lower().split() for each in array:
dic[each] = array.count(each)
print(dic)
this seems to work on python but it's not accepted as a correct solution on teamtreehouse!
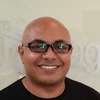
Michael Nessim
7,635 PointsNevermind replace print() with return and it is now acceptable answer.

wads wdsf
Python Web Development Techdegree Student 1,678 Pointsdef word_count(item):
dic={}
item=item.split()
for i in item:
dic[i.lower()]=dic.get(i.lower(),0)+1
return dic

Terry Felder
1,738 PointsMy apologies not sure how to post my code like the rest of you guys...but if anyone please explain why this code isn't accepted.i get no help other than "Bummer: try again " lol. plesae i will use your guys example because i spent 3 days tryna do this on my own it works in my repl.it so just anything would be appreciated
def word_count(my_string): my_string = my_string.lower() dic = dict() for w in my_string.split(): if w in dic.keys(): dic[w] = dic[w]+1 else: dic[w] = 1 return dic

John Napier
14,383 PointsThis worked for me. made 2 lists and did zip :
def word_count(kent):
kent=kent.lower().split()
L=[]
for k in kent:
L.append(kent.count(k))
dkent = dict(zip(kent, L))
return(dkent)

Andrew Kircher
16,612 Pointsdef word_count(string):
string=string.lower()
string_list=string.split(" ")
string_dict={}
for word in string_list:
if word in string_dict:
value= string_dict.get(word)
string_dict[word] = value+1
else:
string_dict.update({word:1})
return (string_dict)
I do not understand why this code does not pass the test
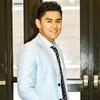
Francisco Ozuna
21,453 PointsDon't forget to split on all whitespace (spaces, tabs, newlines, vertical spaces, etc) like Alexander Davison mentioned above. Currently, you are using .split(" ") which is splitting ONLY on spaces.
use .split() instead of .split(" ")
Ullas Savkoor
6,028 PointsUllas Savkoor
6,028 PointsThanks for the solution, it worked.
Roan Wolfe
10,813 PointsRoan Wolfe
10,813 PointsI wish there were more examples to test with so I could have figured this out on my own, but thanks for explaining that. In future, I will try harder to break my code.
Thanks.
Rahul Kashyap
1,599 PointsRahul Kashyap
1,599 Pointsuhh, davison !! By doing it with above method, why it didn't produce the following output:- {'i': 2, 'i': 2, .......others as given} because in outer for loop it checked 'i' 2 times as the word_list = [] is this ['I' ,'do', 'not' ,'like', 'it', 'Sam' ,'I', 'Am']