Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial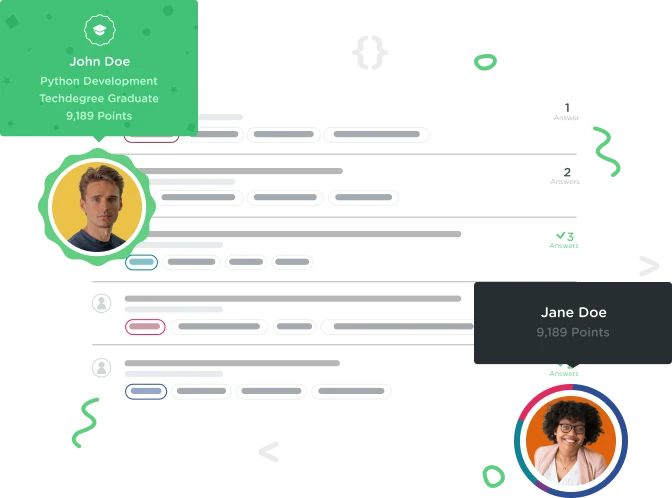

Ammar Fatihallah
7,417 Pointsdictionary output problem
So the other day i did this challenge and wrote the same code and it worked, but i left the courses for a while and came back to it so i can refresh. I wrote this simple code but got no clue why it wouldn't work, any suggestions would be greatly appreciated!
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(tchrs):
for name in tchrs:
return name
max_count = 0
for key in tchrs.values() :
if key > max_count:
max_count = key
return key
2 Answers
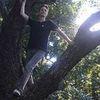
gyorgyandorka
13,811 PointsFirst of all, and probably the most important thing to understand: a return
statement not just returns a specified value (or None
), but exits the function immediately. You can have multiple possible "exit points" (return statements) in your function, but you can only exit once. When you reach a return statement in your function, it means it is the end of that function.
for key in tchrs.values()
--> The naming is confusing here. Since you’re iterating through the values of the dictionary, then it’s logical to name that temporary variable value
. In our case, the dictionary values, which we are iterating through, are lists of courses (of one teacher), so classes
, class_list
, or something like that might be even better (but key
should by no means be used).
We are interested in the number of courses, i.e. the length of those lists:
...
for classes in teachers.values():
if len(classes) > max_count:
max_count = len(classes)
Now we have max_count
, but we’re not finished: we are looking for the name of the teacher, i.e. the corresponding dictionary key. (Remeber that dict[value]
will give back the corresponding key if you have the value.)
Note that we cannot return the name immediately inside the loop, since in that case we would exit the whole function right after the first iteration. So we have to save it in a variable, but this variable (just like max_count
) has to be initialized outside the loop:
def most_classes(teachers):
max_count = 0
name_of_teacher = ""
for classes in teachers.values()
if len(classes) > max_count:
max_count = len(classes)
# teachers[classes] will give back the corresponding key i.e. the name
name_of_teacher = teachers[classes]
return name_of_teacher
This one is not shorter or simpler, but we can also use dict.items()
to iterate throught the keys and values simultaneously:
...
for name, classes in teachers.items()
if len(classes) > max_count:
max_count = len(classes)
name_of_teacher = name
...
The task was only to return the name of the teacher with the most classes, but if you want it, you can return multiple values separated by commas, like this: return name_of_teacher, max_count
. (You can "extract" them in the same order when you call the function.)
...
return name_of_teacher, max_count
name, count = most_classes(teachers)
print("Teacher with most classes: {} ({} classes)".format(name, count))
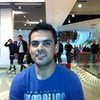
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Ammar
return the teacher i.e the key after you have worked out the max_count. Use dictionary.items() which gives you a bit more flexibility. i changed your code a bit, see below
def most_classes(tchrs):
max_count = 0
teacher = ""
for key,val in tchrs.items() :
if len(val) > max_count:
max_count = len(val)
teacher = key
return teacher

Ammar Fatihallah
7,417 Pointsbut isn't the code suppose to also return the max_count ?
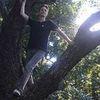
gyorgyandorka
13,811 PointsYou're welcome, glad that it helped to clear up things!
Ammar Fatihallah
7,417 PointsAmmar Fatihallah
7,417 PointsI can't even think of a way to thank you for the great explanation. I really greatly appreciate it