Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial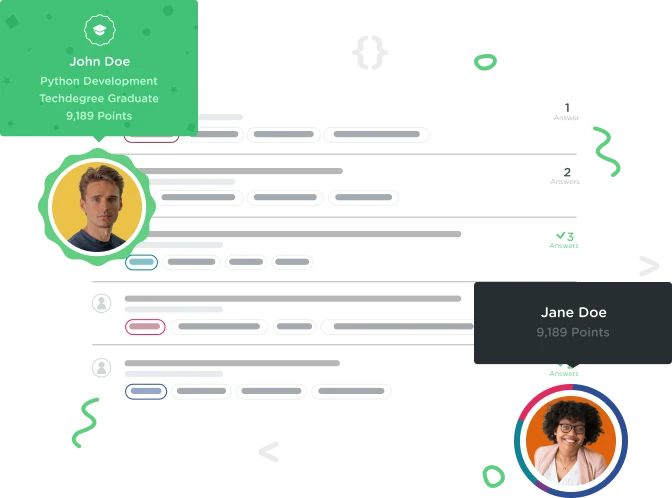

fahad lashari
7,693 PointsDid it in a weird way but it works lol
name = input("Please enter your name: ") number = input("Please enter a number: ")
str_number3 = str(int(number) / 3) str_number5 = str(int(number) / 5)
if int(str_number3[2]) <= 0 and int(str_number5[2]) <= 0: print(number, " is a FizzBuzz")
elif int(str_number3[2]) <= 0: print(number, " is a Fizz number")
elif int(str_number5[2]) <= 0: print(number, " is a Buzz number")
else: print(number, "is neither a Fizzy or Buzzy number")
Just wanted to see if I could this to work before watching the solution
5 Answers
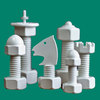
Steven Parker
231,268 PointsI'm wondering how you tested this to get the impression that it worked — it won't actually work for most numbers.
But some hints about creating a workable strategy:
- it's probably easier to work with numbers than strings
- only one conversion will be needed on the input value
- the "remainder" operator ("%") might be handy

fahad lashari
7,693 PointsThe Operator % completely slipped my mind at the time I was doing this lol. I just wanted to try and get something together before watching the solution using only what I've learnt so far.
I do understand that it wont work with most numbers. Thanks for the tips I'll try and get another working version using those.
Regards

fahad lashari
7,693 Points#name = input("Please enter your name: ")
number = int(input("Please enter a number: "))
is_fizz = False
is_buzz = False
if number % 3 == 0 and number % 5 == 0:
is_fizz = True
is_buzz = True
elif number % 3 == 0 and number % 5 >= 1:
is_fizz = True
is_buzz = False
elif number % 3 >= 1 and number % 5 == 0:
is_fizz = False
is_buzz = True
elif number % 3 >= 1 and number % 5 >= 1:
is_fizz = False
is_buzz = False
if is_fizz and is_buzz:
print(number, "is a FizzBuzz")
elif is_fizz and is_buzz != True:
print(number, "is a Fizz")
elif is_fizz != True and is_buzz:
print(number, "is a Buzz")
else:
print(number, " is neither a Fizzy or Buzzy number")
I think this works a lot better.
Thanks for the help!
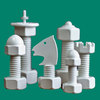
Steven Parker
231,268 PointsMuch better! And you can simplify it a good deal from there by just assigning the booleans directly from the comparison. Then you don't need any conditional statements:
is_fizz = number % 3 == 0
is_buzz = number % 5 == 0

Aaron Wood
2,308 Pointsname = input("Please enter your name: ")
number = int(input("Please enter a number: "))
if(number % 5 == 0):
if(number % 3 == 0):
print("{} is a fizzbuzz number!".format(number))
else:
print("{} is a buzz number!".format(number))
else:
if(number % 3 == 0):
print("{} is a fizz number!".format(number))
else:
print("{} is neither a fizzy or buzzy number.".format(number))

fahad lashari
7,693 PointsThanks! Saw this in the video later. I further simplified it, I didn't know it was possible for calculations or any functionality to run whilst defining the variable. That makes everything so much more interesting lol. Thanks for the help! :)
Simplified code is as follows:
#name = input("Please enter your name: ")
number = int(input("Please enter a number: "))
is_fizz = number % 3 == 0
is_buzz = number % 5 == 0
if is_fizz and is_buzz:
print(number, "is a FizzBuzz")
elif is_fizz and is_buzz != True:
print(number, "is a Fizz")
elif is_fizz != True and is_buzz:
print(number, "is a Buzz")
else:
print(number, " is neither a Fizzy or Buzzy number")